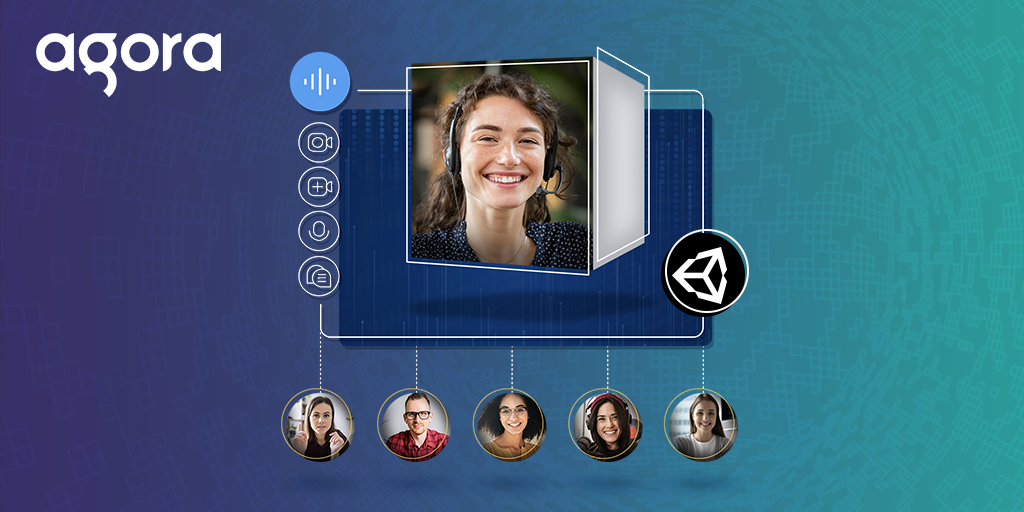
Agora Treefort: Using RTM to Join Breakout Rooms in Unity
Hello, brave developers! In this tutorial you’ll set up a video chat in a 3D Unity environment in Unity 2019.4.1 (LTS) and a messaging feature using the Agora RTM signaling layer. This is essentially a 3D hangout where users communicate with people in the same channel and have the option to create, enter, and share a new channel with users in the RTM network. On joining the scene, each player chooses their username for the network and joins a community LOBBY channel. Each user can select NEW ROOM and create an Agora channel that will show up for joining by other users.
Getting Started with Agora
To get started, you need an Agora account. If you don’t have one, here is a guide to setting up an account.
Here is a GitHub link to the completed project.
Install the Agora SDK from the Unity Asset Store,

And install the RTM SDK Unity package from our GitHub here:

UI Layout
There are two main states for the UI in this tutorial. The first state is an introductory panel where you choose your network username and join the lobby channel. The second state contains a panel that displays all the active channels, an input field to instantiate a new channel, and a logout button used to exit the channel and the RTM network.
First, you’ll create all the necessary UI before coding. Feel free to set up your UI however you’d like, but this is how I made mine, and this is what it looks like:
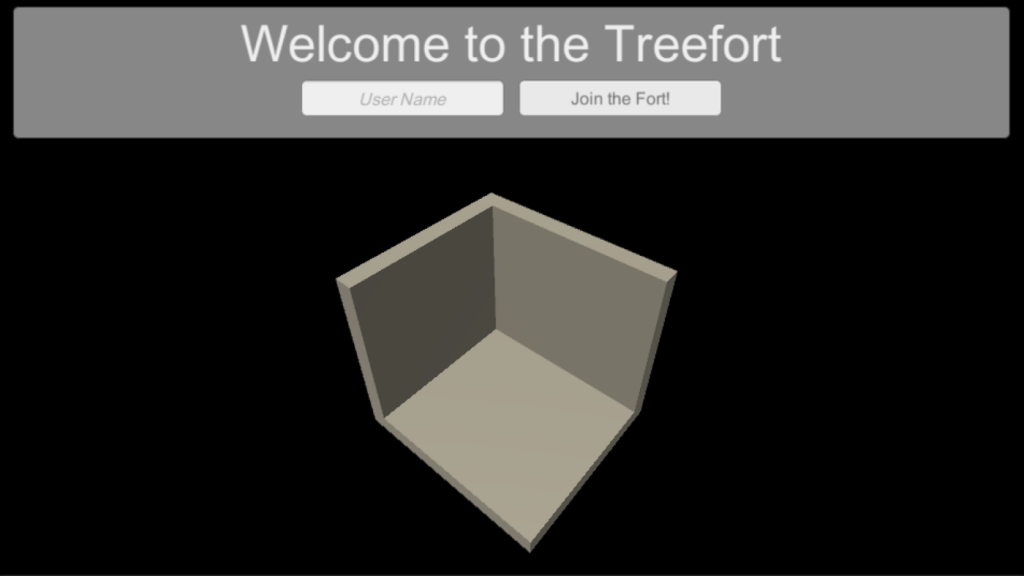
Introduction Panel
Right-click in the Hierarchy window and select UI > Panel. Select the newly created Canvas object. In the Inspector window, set the Canvas > Render Mode to Screen Space — Overlay. Name your newly created panel RTMJoinPanel, and set the Rect Transform values to:
Left: 10
Top: 5
Right: 10
Bottom: 340.
Create a Text object childed to the panel, center it, and enter a welcome message. (I used “Welcome to the Treefort.”) Right-click RTMJoinPanel and select UI > Input Field. Name the new object UserNameInputField, and set its Rect Transform values to:
Pos X: -85
Pos Y: -20
Width: 160
Height: 30.
Right-click RTMJoinPanel and select UI > Button. Name the button JoinTreefortButton, set the button text to “Join the Fort!,” and set its Rect Transform values to:
Pos X: 85
Pos Y: -20
Width: 160
Height: 30.
Channel Panel
Duplicate the RTMJoinPanel to create another panel in the same location, and name the panel ChannelPanel. Rename the duplicated input field to ChannelInputField, the duplicated button to JoinNewChannelButton and the duplicated welcome text to ChannelName. Right-click ChannelPanel and select UI > Dropdown. Last, create a button called LogoutButton. Adjust the X Position of your UI items to your liking, or follow the layout I made here:

This orthographic environment is a series of simple cubes arranged together. There are four spawn points in a square on the floor that mark where the user video cubes will spawn, like so:

Agora Engine
Create an empty GameObject called AgoraEngine in the scene, and attach a script called AgoraEngine.cs. The AgoraEngine will manage the joining and leaving of Agora video channels, and spawning in cubes that render the video from each user.
Helper Tools
I made a simple script called HelperTools.cs to respond if critical objects are null. It’s not crucial to Agora-specific functionality, but if you find the script useful the code is here:
Agora Engine
First, you’ll add the fields and initialize the RTE engine:
Next, we’ll create the join channel functionality and implement the callbacks:
Last, you’ll implement the spawning and deleting of cubes rendering the Agora video data, and an essential cleanup function:
UI Manager
Create an empty GameObject called UIManager in the scene, and attach a script called UIManager.cs. The UIManager is responsible for updating the available active channels, removing inactive channels, and adjusting the UI to ensure that bugs and edge cases are proactively avoided.
First, add the fields and initialize the UIManager:
Next, you’ll implement the functionality that handles the user choosing a channel out of the drop-down list or creating their own channel using the input field name:
In this last section, you implement methods called by the RTMManager when a command is sent from a user through the RTM channel to all other users. When a new channel is added or removed, the network alerts all users and these methods fire:
RtmEngine
The RtmManager is responsible for connecting to Agora’s signaling layer, where you can send text messages through the Agora network. The RTM client is a unique entity and can be used without installing the Agora SDK in your project. In this project, we are using RTM as a pseudo-network that exists outside of the Agora channels. When a user leaves or joins a channel, this action will be communicated to all users in the app, regardless of what channel they are in.
Create an empty GameObject called RtmEngine in the scene, and attach a script called RtmEngine.cs. Next, create the necessary fields and the Start() sequence:
Next, you will implement the functionality placed into buttons to log in and out of the RTM client, and initialize the callbacks referenced in the next section:
In this last section, you implement the RTM-related callbacks and the function to send a message across the RTM channel. When sent, the message is received in the OnChannelMessageReceivedHandler for every user, where they adjust their UI accordingly based on the message:
Editor Setup
Inspector Elements
Now that all the code is written, there will be empty fields in the editor where you need to drag and drop the respective elements into the empty slots. Go through the AgoraEngine, UIManager, and RtmEngine and make sure they are properly filled out.
Here is an example:
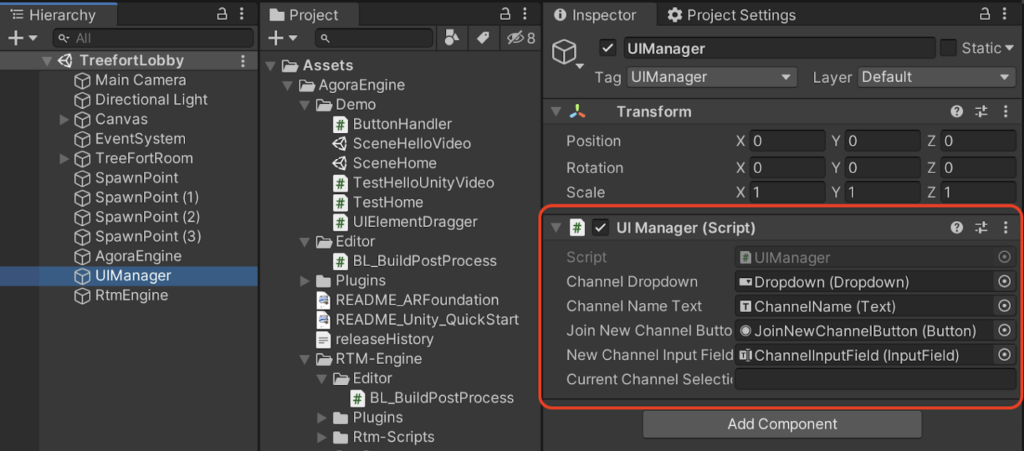
Button Scripts
In the Hierarchy panel, select the JoinTreefortButton, drag the RtmEngine object into the OnClick() event in the button’s Inspector, and select Button_JoinTreefort.
Select the JoinNewChannelButton, drag the AgoraEngine object into the OnClick() event, and select Button_JoinButtonPressed.
Last, select the LogoutButton, drag the RtmEngine into the OnClick() event, and select Button_Logout(), like so:

Dropdown
Click the Dropdown object. In Inspector window > Dropdown Component > Options, add NEW ROOM as the first and only option in the window, like so:

Conclusion
Voila! You’ve now used the Agora RTE and RTM modules to create your own 3D hangout. If you learned something, make sure to tell someone else. And don’t forget to fill in your App ID in the editor!
Here is the GitHub repo where the code is located.
If you are having trouble or want to reach out, you can join the Agora Developer Slack community #unity-help-me.