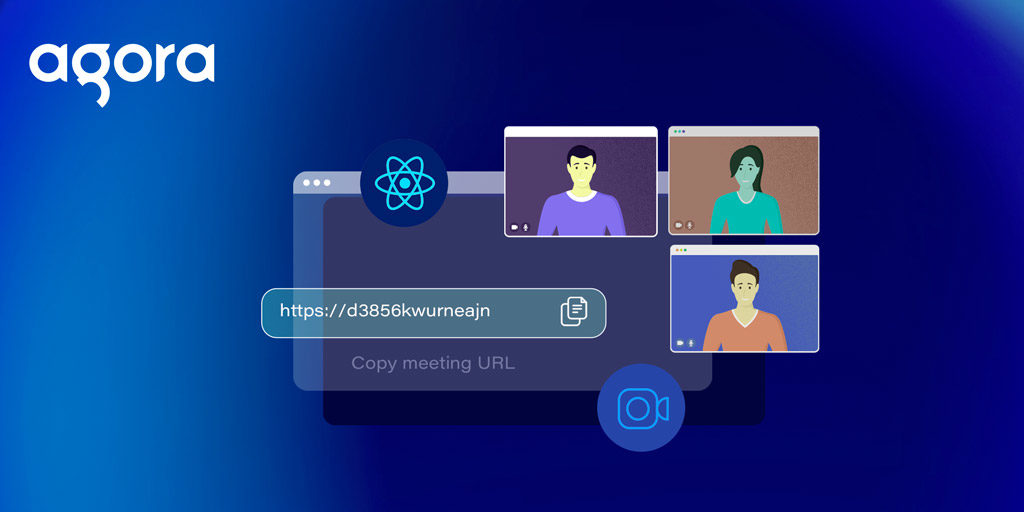
Use Meeting URLs for an Agora Video Call with the React Native UIKit
Update 20-March-22: The blog has been updated to work with v4.0.0 of the Agora React Native UIKit.
The easiest way to join a video call is by sharing a unique link. In this tutorial, we’ll learn how to use universal links with React Native to open up your video calling or livestreaming app built with the React Native UIKit.
To discover more about the React Native UIKit, you can read the release blog or visit GitHub. You can also find the completed project for this blog post here.
Prerequisites
- An Agora developer account (It’s free! Sign up here)
- Node.js LTS release
- Expo CLI (
npm i -g expo-cli
) - Understanding of React Native and Expo
To get us up to speed with a basic boilerplate, I’m using the expo-cli
to create a project using the tabs (TypeScript)
template. You can use the same starting point by executing expo-cli init
and selecting the tabs option when prompted for a template. You can follow the same steps with any app using the react-navigation library.
To use the UIKit with the Expo project, we need to install the expo-dev-client
package (expo install expo-dev-client
) and the Agora React Native UIKit package (yarn add react-native-agora agora-react-native-rtm agora-rn-uikit
).
Using Links with Expo
There are two ways to link to an app: deep links and universal links. Deep links look like this: exp://com.uikit/screen-one?data=hello
. Instead of using the http/https protocol, it begins with a scheme, In the case of Expo apps, the scheme is set to exp
. You can change this to be anything for your app. We’ll use uikit://
.
Universal links are normal web URLs that are supported by your app as well. For example, a URL like https://myapp.com/screen-one?data=hello
can be opened on your website but can also directly open your app if that’s supported. We’ll discuss how to use both deep links and universal links. The Expo docs are a great resource if you’re confused about any of the concepts described here.
Let’s update the app.json
file to define support for our universal link. Let’s take example.com
to be our host name:
"scheme": "uikit",
...
"ios": {
"supportsTablet": true
"supportsTablet": true,
"bundleIdentifier": "com.ekaansh.uikitlink",
"infoPlist": {
"LSApplicationQueriesSchemes": ["uikit"],
"NSCameraUsageDescription": "camera perm",
"NSMicrophoneUsageDescription": "mic perm"
},
"associatedDomains": ["applinks:example.com"]
},
...
"android": {
...
"package": "com.ekaansh.uikitlink",
"intentFilters": [
{
"action": "VIEW",
"data":{
"scheme": "https",
"host": "*.example.com"
},
"category": [
"BROWSABLE",
"DEFAULT"
]
}
]
},
Save the changes and then execute expo prebuild
. This modifies the native projects files (like AndoidManifest.xml
and info.plist
) to add our domain configuration.
Setting up the Domain
AASA configuration for iOS
To implement universal links on iOS, you must first set up verification that you own your domain by serving an Apple App Site Association (AASA) file from your web server. The file should be served from /.well-known/apple-app-site-association
(with no extension). Add appropriate headers to your CDN to ensure that this file is served with the Content-Type=application/pkcs7-mime
header.
An example AASA file looks like this:
{
"applinks": {
"apps": [],
"details": [{
"appID": "<APPLE_TEAM_ID.APP_BUNDLE_ID>",
"paths": ["*"]
}]
}
}
To set up the header for vercel, you can use this example vercel.json file:
{
"headers": [{
"source": "/apple-app-site-association"
"headers" : [{
"key" : "Content-Type",
"value" : "application/pkcs7-mime"
}]
}]
}
Visit the Apple documentation for more details on the format of the AASA.
Verify the Domain for Android (Optional)
By default, you’ll see the select app dialog box when using your universal link. If you want your link to always open your app without presenting the select app dialog box, you must publish a JSON file at /.well-known/assetlinks.json
specifying your Android App ID and which links should be opened by your app. See the Android documentation for details.
Setting Up Linking
In the navigation/LinkingConfiguration.tsx
file, we’ll update the prefix to support the universal link:
prefixes: [‘https://example.com', Linking.createURL(‘/’)]
This lets the app router handle both universal links and deep links.
Let’s modify the second screen (./screens/TabTwoScreen.tsx
) to open the UIKit. We’ll store the channel as a state variable. We’ll add a listener for the URL where we extract the channel from the query and update our state:
Next, we’ll create a useEffect
hook to get the channel from the query when the app is opened from a universal link. We’ll use the getInitialUrl
and parse
methods to extract the channel and update the state:
Finally, we can render the UIKit. You can read more about how to set this up here. Make sure to add your own App ID to the rtcProps
:
To open the UIKit screen from within the app, we can use Linking
from expo and call the deeplink uikit://two?channel=test
. I’ll add a <Text>
component to navigate to the UIKit in ./screens/TabOneScreen.tsx
:
Bonus: Generating Links
A quick way to generate unique links is by using the current timestamp using the Date
object in JavaScript. Here’s a simple function to create a new room:
Conclusion
That’s a painless way (comparatively speaking) to set up universal links to work in Android and iOS using React Native and Expo. You can read the Expo and React Navigation docs to learn more.
If you have questions while using the Agora React Native UIKit, I invite you to join the Agora Developer Slack community, where you can ask them in the #react-native-help-me
channel. Feel free to open issues for feature requests or to report bugs on the GitHub Repo.