
Recording Live Streams with an On-Prem Recording Service with C++ and Docker
Live streaming apps are growing in popularity and one of the most requested features is recording the streams for later use.
Today we’ll go through the steps to build a Dockerfile
to easily deploy an Agora Recording Server within your backend orchestration.
Pre-Requisites
- A basic understanding of Docker
- Docker locally installed
- A developer account with Agora
- An Agora based live streaming or communications app
Setup Project
Let’s start by opening our favorite code editor and creating our Dockerfile
.

We’ll set our container to use the latest Ubuntu image as its base, run apt update
to get all updates, and then we’ll add the commands to install our dependencies.
# use latest ubuntu base image
FROM ubuntu:latest
RUN apt update
# install any needed packages/tools
RUN apt install -y git
RUN yes | apt-get install build-essential
RUN apt-get install gcc
For debug purposes, let’s also add in support for core dumps.
RUN ulimit -c unlimited
Next we will download the Agora Linux SDK and unzip it to the root directory.
# download agora sdk zip
ADD https://download.agora.io/ardsdk/release
/Agora_Recording_SDK_for_Linux_v2_3_4_FULL.tar.gz
# unzip sdk
RUN tar xxzf Agora_Recording_SDK_for_Linux_v2_3_4_FULL.tar.gz
Once the SDK is unzipped, we’ll need to switch the working directory to samples/cpp
and run make
# go to c++ sample directory
WORKDIR Agora_Recording_SDK_for_Linux_FULL/samples/cpp
# run make
RUN make
Now we need to make sure we have an Agora AppID, because the Recording Server will need to use it along with the Channel name to join the channel.
# run recording server
CMD ./recorder_local --appId <YOUR_APPID> --uid 0 --channel demo
--channelProfile 0 --appliteDir ../../bin
Note: there are two channelProfiles: 0=Communication, 1=Live broadcast
Lastly we’ll expose the necessary ports.
# receiving ports
EXPOSE 4000/udp
EXPOSE 41000/udp
EXPOSE 1080/tcp
EXPOSE 8000/tcp
EXPOSE 4001-4030/udp
EXPOSE 1080/udp
EXPOSE 8000/udp
EXPOSE 9700/udp
EXPOSE 25000/udp
Now we are ready to build and run our container.
docker build -t agorarec . && docker run -it agorarec

Now using our Agora Live Streaming web or native app, we can test the recording. If you don’t have an app built or want to test quickly for this tutorial, we can use the group chat web app (https://agora-group-video-chat.herokuapp.com) that we previously built together. Once the first user enters the room, you should see the output from the container in your console.

When testing with 3 streams, your logs should look similar to those below.
User 2199979035 joined, RecordingDir:./20190918/
demo_225632_937626000/
onFirstRemoteAudioFrame,User 2199979035, elapsed:331
User 1503933393 joined, RecordingDir:./20190918
/demo_225632_937626000/
onFirstRemoteAudioFrame,User 1503933393, elapsed:339
pre receiving video status is 0 now receiving video status is 1
pre receiving audio status is 0 now receiving audio status is 1
User 4190354927 joined, RecordingDir:./20190918
/demo_225632_937626000/
onFirstRemoteAudioFrame,User 4190354927, elapsed:18291
User 4190354927 offline, reason: 0
User 2199979035 offline, reason: 0
User 1503933393 offline, reason: 0
pre receiving video status is 1 now receiving video status is 0
pre receiving audio status is 1 now receiving audio status is 1
stopre receiving video status is 0 now receiving video status is 0
pre receiving audio status is 1 now receiving audio status is 0
Next Steps
If you’d like to compare Dockerfiles
😉, you can scope mine below.
The last step would be to deploy this Dockerfile to your cloud services provider to be triggered by a Serverless function or possibly an always-on service. The choice is up to you!
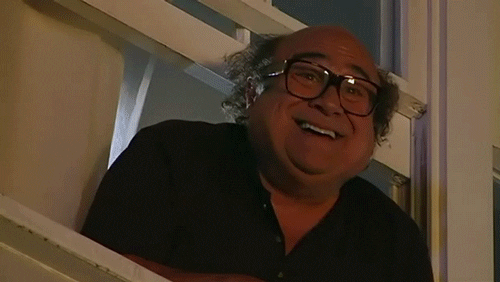
For more information about the Agora On-Prem Recording SDK, please refer to the Agora On-Prem QuickStart and API Reference.