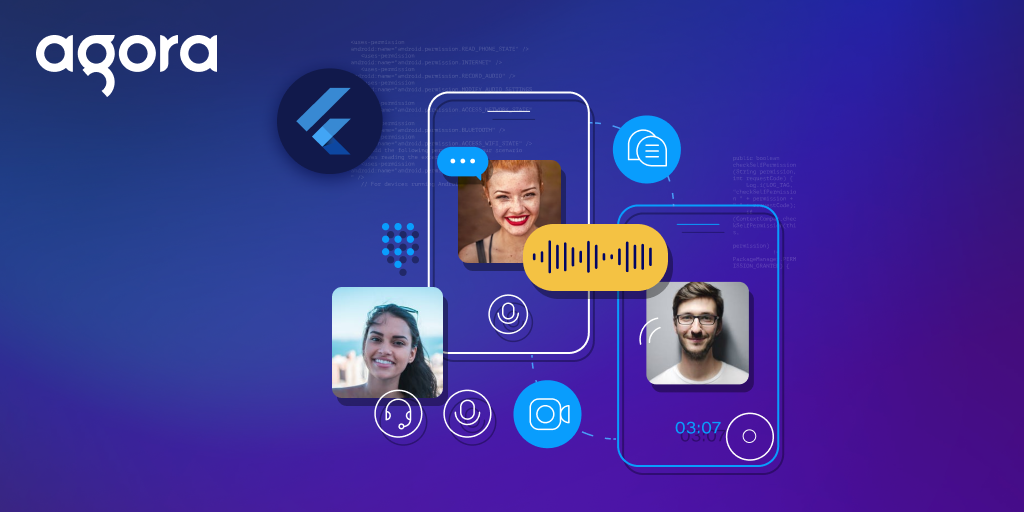
Real-Time Messaging and Video with Dynamic Channels Using the Agora Flutter SDK
Yesterday, I was dying of boredom while attending my Chemistry lecture, so I started looking at the software my college is using for their lectures. In this software, they list out a bunch of subjects per user and then display how many students are currently attending lectures for that particular subject.
This intrigued me a bit as to how easily a person can create a channel and then others can join that channel to interact with the host. So I thought of making a clone of this software using the Agora Flutter SDK. If you have seen our quickstart guide, then you know that both users need to enter the same channel name to get on a call. This creates a problem because the user can’t share the channel once it has been created without a proper back end.
To avoid going through that complex back-end route where we maintain a database of all the users and the channels they create, I leveraged the Agora RTM SDK to simplify the process. This way when a user joins, every host sends out a message with their channel name along with the number of users in it.
So grab a cup of coffee and let’s get started!
Prerequisites
- An Agora developer account (see How to get started)
- Flutter SDK
- VS Code or Android Studio
- A basic understanding of Flutter development
Project Overview
- A user logs in to the RTM channel named lobby using a unique username. This channel is used to display all the preexisting channels and to signal the user as new channels are created.
- So instead of asking all the users present in the lobby to share the channel details, we just ask the host or the oldest member in the channel to share the details.
- The message is sent in the form of channelName:memberCount. Once this message is received, we map the channel name to the member count. This map is then used to display the list of active channels.
- A user clicks one of the active channels, and then all the users in that channel are taken to a group video call.
Project Setup
- We begin by creating a Flutter project. Open your terminal, navigate to your dev folder, and enter the following:
flutter create agora_dynamic_channels
2. Navigate to your pubspec.yaml
file. In that file, add the following dependencies:
Be careful with the indentation when adding the packages because you might get an error if the indentation is off.
3. In your project folder, run the following command to install all the dependencies:
flutter pub get
4. Once we have all the dependencies, we can create the file structure. Navigate to the lib folder and create a file structure like this:
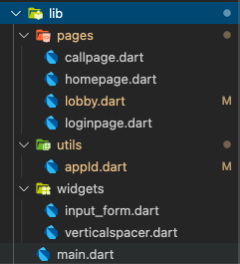
Building the Login Page
The login page is pretty simple: we just add an input field for the username and a submit button. This channel name is then shared with the next page — lobby.
This will create a page like this :
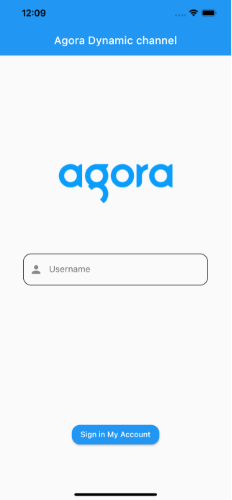
Building the Lobby
We initialize our lobby by making all the users join a common RTM channel named lobby. We use this channel to share the details of the channel that a user creates.
The following steps occur in a user journey at the lobby page:
- The Agora RTM channel is initialized to join the channel named lobby.
- The user receives messages from the other users in the lobby channel about the channel details.
- The user either creates a channel of their own or subscribes to a preexisting channel. If the user joins a preexisting channel, then we just send a message to the host, which in turn increases the user count and shares it with the lobby. Or if the user decides to create their channel, then we create another object of RTM Channel and share the channel details to all the users in the lobby.
- Based on either of the user actions, the user is redirected to the callpage.
That might be a lot to register, so let’s break it down:
_createClient()
: This function is triggered as soon as you join the page so that a user can join the RTM channel named lobby. It also listens to the broadcast message from the hosts to get a list of active channels along with the user count in those channels._createChannel()
: This function listens to event handlers to maintain a list of active users and channels._createChannels()
: This function is used when a user plans to create their channel instead of joining an existing channel._joinCall()
: This function is used to navigate to the call screen. But before that, it creates another object of the RTM channel, which is used to join the selected channel. This way the user can join this subchannel while still being connected to the lobby channel.
This will give you a page similar to this:
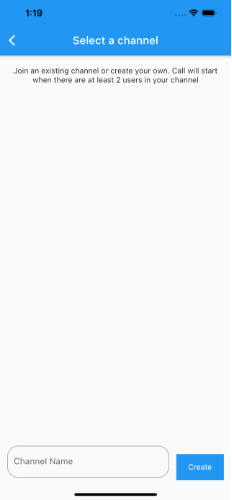
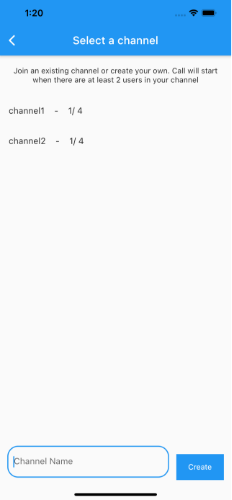
Building the Video Chat Page
Here, we create a simple group video chat application using the Agora RTC SDK. All the users who selected the same channel name are grouped here in the same call.
We initialize the AgoraRtcEngine with the App ID. The object of this method is then used to call other functions required to set up the call.
We then create a function to manage all our event handlers, which are triggered when a user joins a channel or leaves a channel. This helps in maintaining a list of UIDs. We use this list to render video feeds.
A user then joins a particular channel using the joinChannel()
method. In the joinChannel()
method, you get the option to use tokens along with the UID and the channel name.
Note:This project is meant for reference purposes and development environments. It is not intended for production environments. Token authentication is recommended for all RTE apps running in production environments. For more information about token-based authentication in the Agora platform, see this guide: https://bit.ly/3sNiFRs
Once, you have implemented the above given code, you will have a screen similar to this:
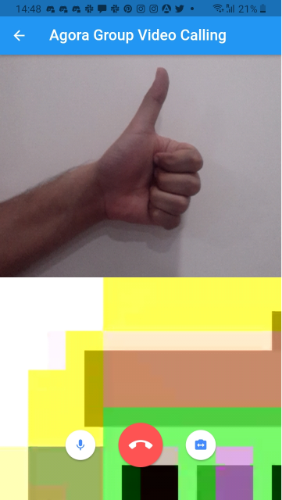
Conclusion
Awesome! You have implemented a video chat application with dynamic channels built using the Agora Flutter SDK. In this app, the superuser is selected depending upon who created the channel. This user shares the details of the channel with others. This functionality makes the application scalable.
You can get the complete code for this application here.

Other Resources
To learn more about the Agora Flutter SDK and other use cases, you can refer to the developer guide given here.
You can also have a look at the complete documentation for the functions discussed above and many more here.
And I invite you to join the Agora Developer Slack Community.