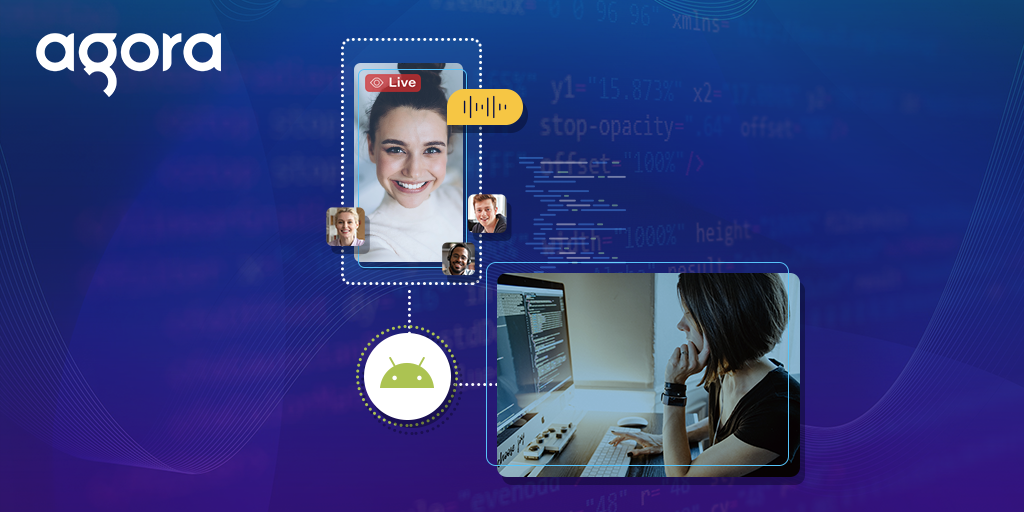
Creating an Android Video Streaming Application with Three Lines of Code
Agora UIKit for Android Makes Video Streaming Easier Than Ever
When you’re setting up your own streaming application with Agora, a few technical steps might slow you down. Now with Agora UIKit for Android, you can create an application as easily as placing a view in your app’s
Content
- Prerequisites
- Setup
- Adding Video Streaming to Your App
- Default Functionality
- Basic Customization with AgoraSettings
- Advanced Customization with Agora Video Viewer
- AgoraSingleVideoView
- Conclusion
- Other Resources
Prerequisites
- An Agora developer account (see How to Get Started with Agora)
- Android Studio
- An Android device running Android 24+
- A basic understanding of Android development
Setup
Create an Android application with Android Studio, then open your root build.gradle
and add jitpack to the end of repositories:
allprojects {
repositories {
...
maven { url 'https://www.jitpack.io' }
}
}
Add the Agora UIKit for Android dependency:
dependencies {
implementation ‘com.github.AgoraIO-Community:Android-UIKit:v2.0.0’
}
After you sync your gradle files, the module should be installed and ready to use.
Add all the required permissions to your AndroidManifest.xml
:
<manifest>
...
<uses-permission android:name="android.permission.READ_PHONE_STATE"/>
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<!-- The Agora SDK requires Bluetooth permissions in case users are using Bluetooth devices.-->
<uses-permission android:name="android.permission.BLUETOOTH" />
...
</manifest>
Adding Video Streaming to Your App
In this module, the main class type is AgoraVideoViewer. To create a basic instance of this class, you only need your Agora App ID ready:
val agView = AgoraVideoViewer(
this, AgoraConnectionData(“app-id-here”)
)
From here, place the view in your activity. In this example, it is going to fill the entire screen:
this.addContentView(
agView,
FrameLayout.LayoutParams(
FrameLayout.LayoutParams.MATCH_PARENT,
FrameLayout.LayoutParams.MATCH_PARENT
)
)
Finally, join a channel, and let AgoraVideoViewer
figure everything else out:
agView.join(“test”)

Default Functionality
By default, Agora UIKit for Android includes the following functionality before any customization:
- Automatically laying out all video streams
- Displaying the active speaker in the larger display in the floating layout
- Allowing you to pin any stream to the larger display in the floating layout
- Buttons for disabling the camera or the microphone and switching cameras
- An icon for signalling that the local or remote streamer’s microphone is disabled
- Automatically subscribing to high- or low-quality video streams, depending on the layout
- A choice of two layouts: floating and grid
Basic Customizing with AgoraSettings
A few basic and more advanced features in this library make it customisable for your needs. And there’s a growing list of things to customise using AgoraSettings.
Positioning Elements
The position of the collection view of all the streamers when using floating layout and the collection of buttons (such as mute microphone and sw) can be moved around with floatPosition
and buttonPosition
, respectively. You have the choice of top, right, bottom and left for this enumeration. floatPosition
defaults to the top, and buttonPosition
defaults to the bottom.
Colors
The colors that can be changed are found in AgoraSettings.colors
, and that property is of type AgoraViewerColors
.
The available colors are micFlag, floatingBackgroundColor, floatingBackgroundAlpha, buttonBackgroundColor, and buttonBackgroundAlpha. The following image is annotated to show all of those:
var agSettings = AgoraSettings()
agoraSettings.colors.micFlag = Color.RED
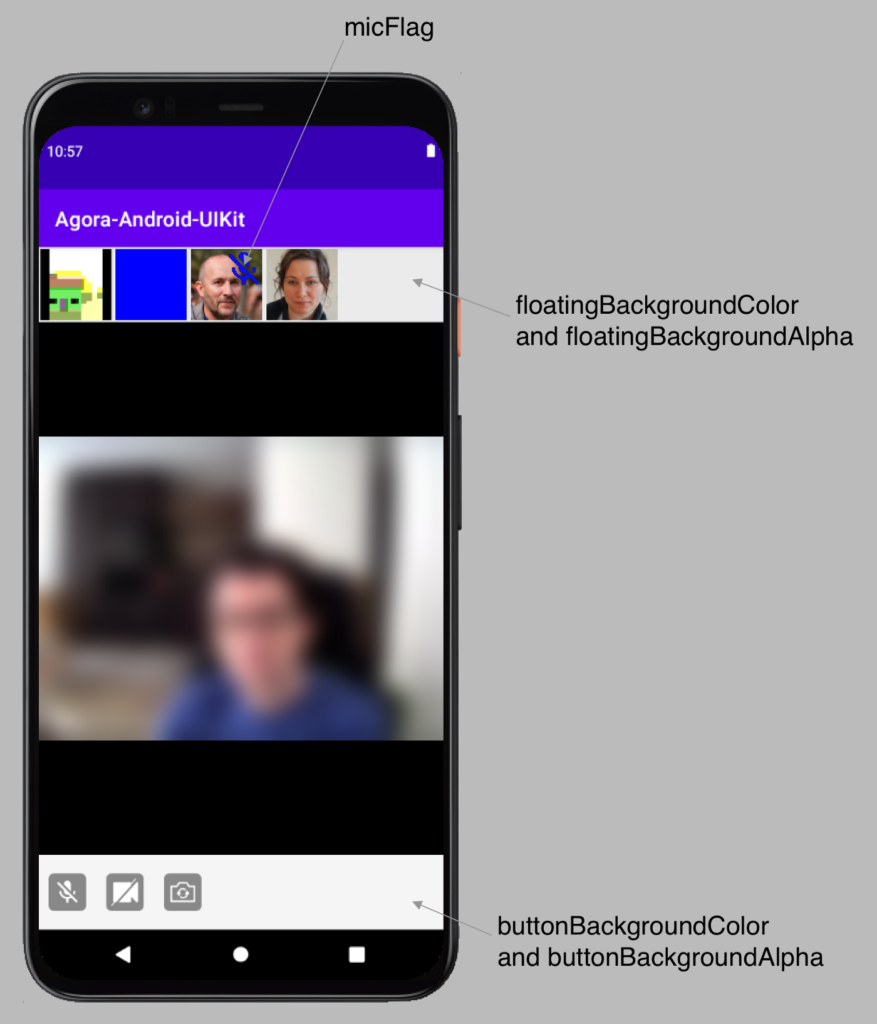
Choosing the Button Set
By default, all the buttons are added (enable/disable camera, switch camera, mute/unmute microphone). The set of buttons is created using the parameter enabledButtons. enabledButtons is a mutable list of enum
, of BuiltinButton
type.
If you want to show only the button to enable and disable the camera, you could set enabledButtons like this:
val agoraSettings = AgoraSettings()
agoraSettings.enabledButtons = mutableSetOf(AgoraSettings.BuiltinButton.CAMERA)
Or if you want to show the camera, microphone, and switch camera buttons:
val agoraSettings = AgoraSettings()
agoraSettings.enabledButtons = mutableSetOf(
AgoraSettings.BuiltinButton.CAMERA,
AgoraSettings.BuiltinButton.MIC,
AgoraSettings.BuiltinButton.FLIP
)
Then apply these settings using the AgoraVideoViewer initialiser:
agView = AgoraVideoViewer(
this, AgoraConnectionData(“app-id-here”),
agoraSettings=this.settingsWithEnabledButtons()
)
val set = FrameLayout.LayoutParams(FrameLayout.LayoutParams.MATCH_PARENT, FrameLayout.LayoutParams.MATCH_PARENT)
this.addContentView(agView, set)
agView!!.join(“test”)
In the Advanced Customization section below, you see how to add your own custom button.
Video Settings
You can find a few parameters in AgoraSettings to change the way the video stream looks.
AgoraSettings.videoRenderMode lets you choose the AgoraVideoRenderMode for all the videos rendered on your local device, with a choice between fill, fit, and hidden. See the full documentation on AgoraVideoRenderMode here.
AgoraSettings.videoConfiguration lets you add your own AgoraVideoEncoderConfiguration, with options for size, frame rate, bitrate, orientation, and more. See the full documentation for AgoraVideoEncoderConfiguration here.
You can also customize settings for dual-stream mode in several ways. By default, a session created with AgoraVideoViewer enables dual-stream mode and adopts a default low-bitrate parameter identical to the one shown in the example below. This can be customized by setting AgoraSettings.lowBitRateStream, like this:
val agoraSettings = AgoraSettings()
agoraSettings.lowBitRateStream = """
{
“che.video.lowBitRateStreamParameter”: {
“width”:160,”height”:120,”frameRate”:5,”bitRate”:45
}
}
"""
To better understand this setting, the following post talks in depth about dual-stream mode and the low-bitrate stream parameter:
Video for Multiple Users — In a video call or interactive video streaming scenario, if multiple users publish video streams at the same time, user…
With AgoraVideoViewers, all videos in the floating view collection are subscribed to the low-bitrate stream until they move to the main view or the layout changes to grid mode. The grid mode may also subscribe to the low-bitrate stream if the number of videos in the grid passes the threshold value, which is found in AgoraSettings.gridThresholdHighBitrate.
This value can be set to any integer. The default value is 4. This means that if 5 videos are shown in the grid view, then they are all subscribed to the low-bitrate feed.
Subscribing to the low-bitrate mode is important for a couple of reasons: It reduces the bandwidth used by all users subscribed to the feed. And it can help avoid using more minutes on your Agora account by reaching a higher-streaming band.
Token Fetching
The final parameter currently in this library is AgoraSettings.tokenURL. This property is an optional string that can be used to automatically fetch a new access token for the channel if the provided token has expired or is due to expire soon.
The functionality for requesting a new token expects the token to follow the URL scheme in the Golang token server found on GitHub: AgoraIO-Community/agora-token-service.
To set up this property, you can add the following code, replacing the address with the URL path to your own token server:
val agoraSettings = AgoraSettings()
agoraSettings.tokenURL = “http://10.0.2.2:8080"
This feature can be triggered when calling the join
method on your AgoraVideoViewer, like this:
agView!!.join("test", role = Constants.CLIENT_ROLE_BROADCASTER, fetchToken = true)
Any attempt to fetch a new token will be triggered only if a tokenURL is provided in the AgoraSettings for this AgoraVideoViewer object.
Advanced Customization of AgoraVideoViewer
Selecting a Default Layout — Grid or Floating
You may want to style your video view in a form of either Grid or Floating. You can use the Style method for that. To use a Grid layout, you must set a style to the AgoraVideoViewer
class:
agView = AgoraVideoViewer(
this, AgoraConnectionData(“app-id-here”)
)
agView!!.style = AgoraVideoViewer.Style.GRID
You can similarly create a Floating view like this:
agView = AgoraVideoViewer(
this, AgoraConnectionData(“my-app-id”)
)
agView!!.style = AgoraVideoViewer.Style.FLOATING
Adding More Buttons with AgoraVideoViewerDelegate
You may want to add your own buttons to the enabledButtons. You can use the delegate method extraButtons for that. To use extraButtons, you must set a delegate to the AgoraVideoViewer. There is a parameter for this in the AgoraVideoViewer initialiser.
An example implementation of extraButtons might look like this:
val agoraSettings = AgoraSettings()
val extraButton = AgoraButton(this)
extraButton.setImageResource(android.R.drawable.star_off)
agoraSettings.extraButtons = mutableListOf(extraButton)

This code adds a button with the star image android.R.drawable.star_off. It is added to the end of the button collection, adopting the same style as the other buttons in the collection.
You can add a bit of functionality to the button in the exact same way you would any other button, by using clickAction:
val agoraSettings = AgoraSettings()
val extraButton = AgoraButton(this)
extraButton.clickAction = {
it.isSelected = !it.isSelected
extraButton.setImageResource(if (it.isSelected) android.R.drawable.star_on else android.R.drawable.star_off)
it.background.setTint(if (it.isSelected) Color.GREEN else Color.GRAY)
}
extraButton.setImageResource(android.R.drawable.star_off)
agoraSettings.extraButtons = mutableListOf(extraButton)
return agoraSettings

Since the buttons are being added to a set of existing buttons, I advise against customising these buttons too much, to avoid the risk of the design falling apart. If you wish to add completely custom buttons, the best way is to add them on top of the AgoraVideoViewer object and set the enabledButtons setting to an empty set.
AgoraSingleVideoView
As well as displaying all the video views together, you may want to place and organise them independently. You can do this with AgoraSingleVideoView.
This is the same way that AgoraVideoViewer displays the boxes. But if you want to create the views yourself for a remote user, you can do so like this:
override fun onJoinChannelSuccess(channel: String?, uid: Int, elapsed: Int) {
super.onJoinChannelSuccess(channel, uid, elapsed)
val vidView = AgoraSingleVideoView(this.context, 0, Color.BLUE)
// agoraEngine is a reference to your active RtcEngine
agoraEngine.setupLocalVideo(vidView.canvas)
// position the vidView in your scene…
}
The documentation for AgoraSingleVideoView can be found here.
Conclusion
If there are features you think would be good to add to Agora UIKit for Android that many users would benefit from, feel free to fork the repository and add a pull request. Or open an issue on the repository with the feature request.
The plan is to grow this library and have similar offerings across all supported platforms. There are already similar libraries for React Native and iOS, so be sure to check those out.
Other Resources
For more information about building applications using Agora SDKs, take a look at the Agora Video Call Quickstart Guide and Agora API Reference.
And I invite you to join the Agora Developer Slack community.
Build an Android video call app with only 3 lines of code
