Creating a video call app is super straightforward with the Agora SDK and Agora UIKit. But what about adding cool features to enhance the overall user experience or adding monetisation options? That’s where the Agora Extensions Marketplace comes in.
The Extensions Marketplace launched in September 2021, and already several extensions are available, with more to come.
In this post, we’ll show you how to remove background noise from your existing video call app with the Bose PinPoint extension. With PinPoint, you can get rid of background noise in just two easy steps:
- Enable the extension.
- Pass extension credentials.
Now, let’s get started.
Prerequisites
- An Agora developer account — Sign up here
- Xcode 12.3 or later
- A physical iOS device with iOS 13.0 or later
- A basic understanding of iOS development
Setup
Let’s start with a new, single-view iOS project. Create the project in Xcode, choosing SwiftUI for the interface, and then add the Agora UIKit package.
Add the package by selecting File > Swift Packages > Add Package Dependency
, and then paste the link into this Swift package:
https://github.com/AgoraIO-Community/iOS-UIKit.git
When asked for the version, insert 4.0.0-preview
, and select "Up to Next Major". This should install at least version 4.0.0-preview.8
, which is released at the time of writing.
When in doubt, you can always select “Exact” and insert 4.0.0-preview.8
.
Once that package is installed, the camera and microphone usage descriptions need to be added. To see how to do that, check out Apple’s documentation here:

Video Call UI
I won’t go into much detail about the UI here, because we’re mainly focusing on the PinPoint extension, but on a high level:
- The ContentView contains two views: an AgoraViewer (defined in Agora UIKit) and a button to join the channel.
- When the join button is pressed, the Agora UIKit method
join(channel:,with:,as:)
is called, which joins the Agora video channel.
This is what the ContentView looks like:
The AgoraViewerHelper
helper object, without any of the PinPoint additions, looks like this:
We now have a working video call app with SwiftUI and Agora UIKit. The next step is to integrate the PinPoint extension!
Integrating PinPoint
Credentials
If you have an account with Agora and are currently signed in, follow this link to activate the extension for your account.
First, you will need to activate PinPoint for your account by clicking “Activate” here:
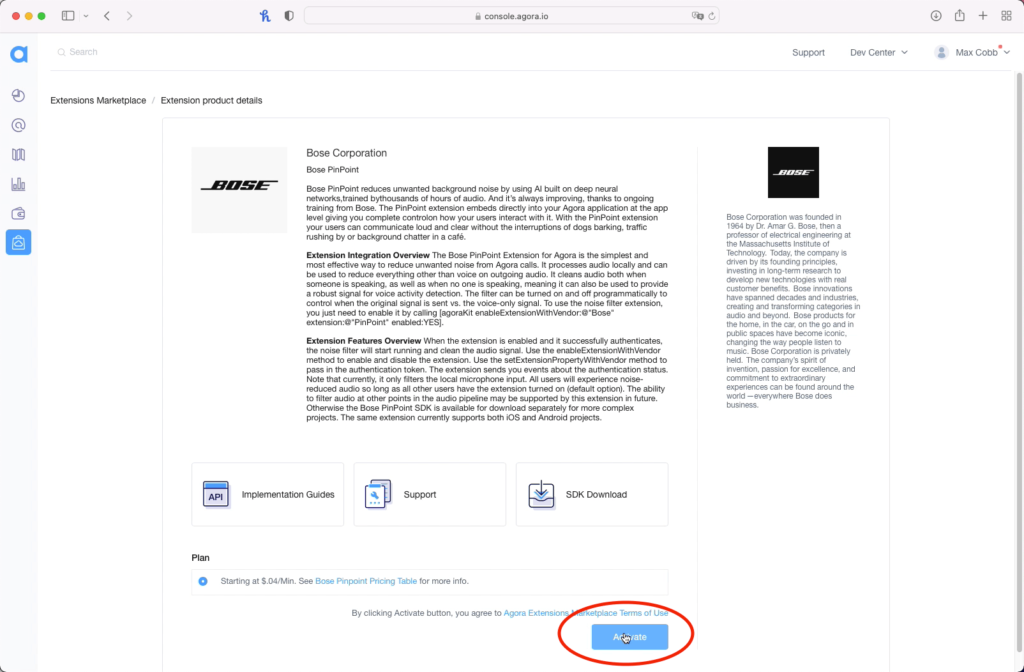
Next, enable PinPoint for the project, the same project as for the Agora App ID used in the app:
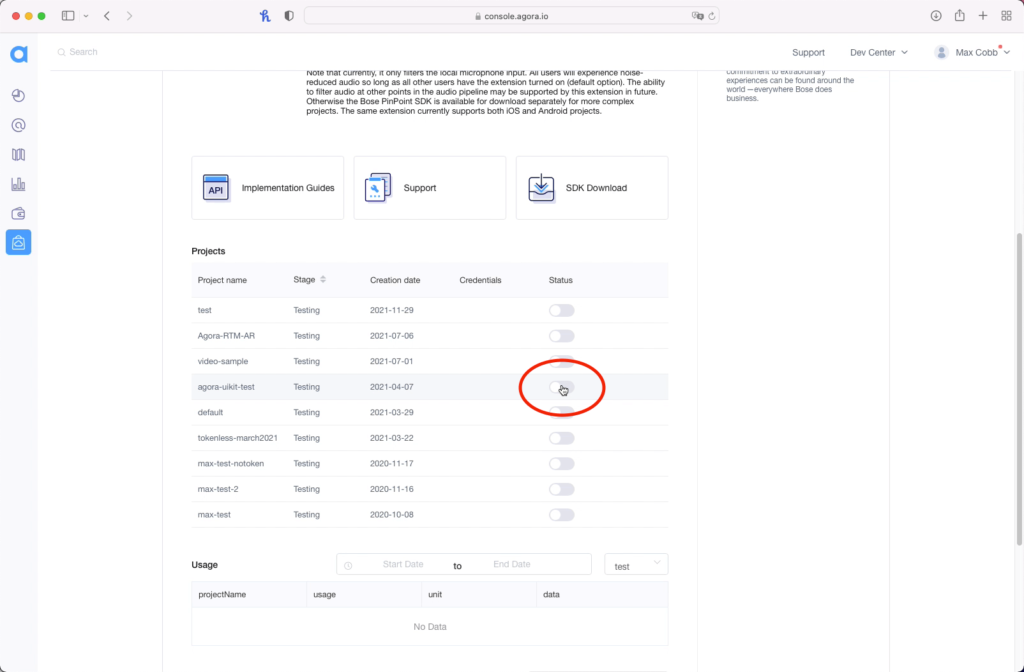
Once that’s done, you can grab your PinPoint API Key
and API Secret
by clicking “view” in the Credentials column:
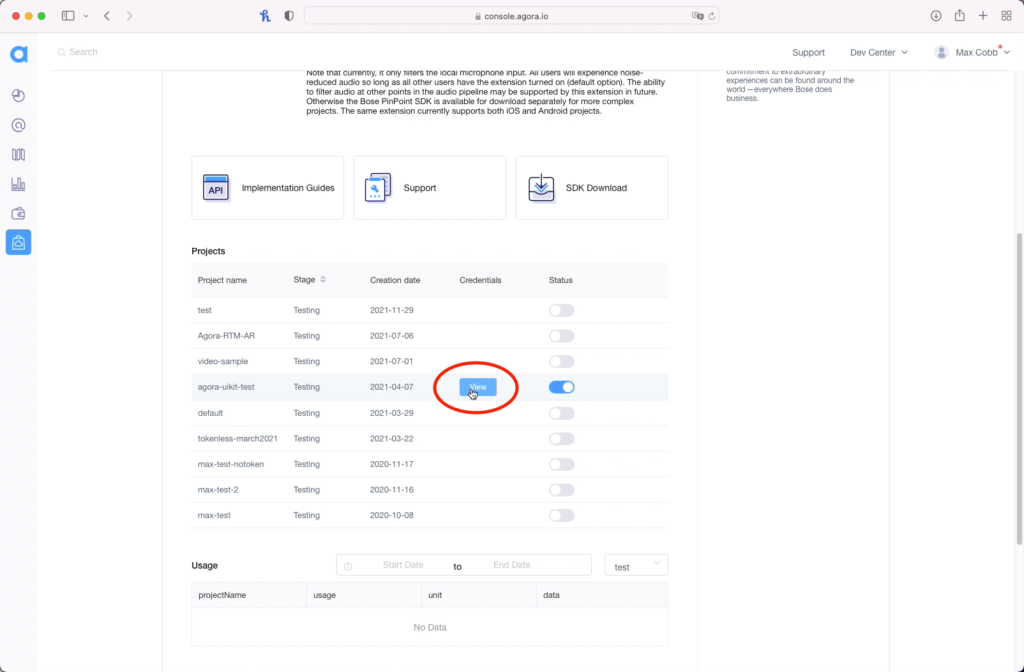
Add to Xcode
We need to add the extension, which can also be installed via the Swift Package Manager with the following URL:
https://github.com/AgoraIO-Community/Extension-Bose-PinPoint-iOS.git
The latest release at the time of writing is 0.0.1
.
This package doesn’t need importing into your Swift code. It just needs to be bundled alongside the Agora SDK in your app target.
Enable the Extension
When using Agora extensions, you need to enable them before joining a channel.
To do this with Agora UIKit, call AgoraVideoViewer.enableExtension()
.
This method needs to be passed the vendor (“Bose”) and the vendor extension (“PinPoint”) in this case:
AgoraViewerHelper.agview.viewer.enableExtension(
withVendor: "Bose", extension: "PinPoint", enabled: true
)
The above code snippet is added to the beginning of the joinChannel
method defined earlier.
Toggle the Extension
As shown in the above snippet for AgoraViewerHelper, we have added an extra button to the default buttons provided by Agora UIKit.
This button displays the PinPoint logo and toggles between a green and a red background. When clicked, the button calls a method togglePinpoint
. Let’s link up this button to enable and disable the extension:
@objc func togglePinpoint(_ sender: UIButton) {
pinPointEnabled.toggle()
sender.backgroundColor = self.pinPointEnabled ?
.systemGreen : .systemRed
AgoraViewerHelper.agview.viewer.enableExtension(
withVendor: "Bose", extension: "PinPoint",
enabled: self.pinPointEnabled
)
}
In the above snippet, all we need to add is the call enableExtension again, with the enabled
property toggling between true
and false
.
Almost there now! The only thing left to do is define the registerPinPoint
method.
This method needs to pass the API Key and API Secret values. These values need to be passed to the extension with the keys apiKey
and apiSecret
, respectively:
func registerPinPoint() {
// Set API Credentials
AgoraViewerHelper.agview.viewer.setExtensionProperty(
"Bose", extension: "PinPoint",
key: "apiKey", value: AppKeys.pinPointApiKey
)
AgoraViewerHelper.agview.viewer.setExtensionProperty(
"Bose", extension: "PinPoint",
key: "apiSecret", value: AppKeys.pinPointApiSecret
)
}
Conclusion
You now have a video call app complete with the new extension from Bose!
There are other extensions I’d encourage you to try out. They can all be found here:

If you want to create an app from scratch with this extension, the extension can be applied to an application made directly with the Agora SDK. For that, you will need to make sure you’re using the 4.x version of the SDK. Details can be found here:

The methods, such as setExtensionProperty
, used in this post are adaptations of the SDK's built-in methods relating to extension. They can be found in this file from the Agora Video UIKit:

Testing
You can try out this app by following the GitHub link:

Other Resources
For more information about building applications using Agora SDKs, take a look at the Agora Video Call Quickstart Guide and Agora API Reference.
I also invite you to join the Agora Developer Slack community to meet with our developer team as well as other like-minded developers and technical enthusiasts.