Security within video chat applications is necessary now that remote working and virtual events are part of the workplace. In the Agora platform, one good way to add a layer of security on your stream is to add a token service. A token is a dynamic key that is generated using a set of given inputs. The Agora platform uses tokens to authenticate users.
In this tutorial, you learn how to fetch an Agora token from a web service running an Agora token server, using Unity and C#.
To jump straight to a full working example, see the following repository:
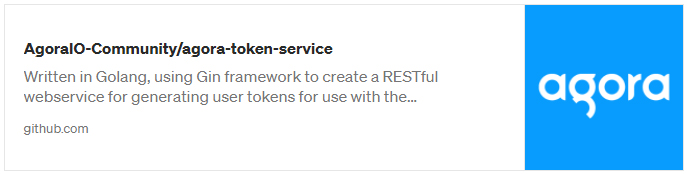
The project in this repository with the token example is video-sample-token.
Prerequisites
- An Agora developer account (see How To Get Started with Agora)
- A basic understanding of C# and Unity
- Unity
- An Agora token server, either local or remote (see Agora Token Service)
Project Setup
Create a Unity project by following the steps in the Quickstart Guide, or go straight to the example project on GitHub.
A previous article on how to create a token server can be found here. To quickly launch a token server, this GitHub repository has all the necessary code laid out:
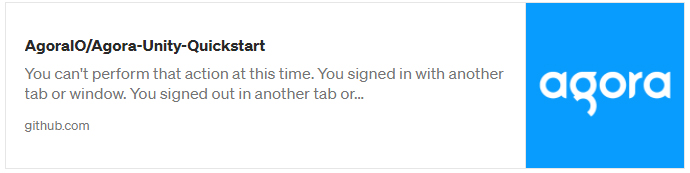
When you have your token server set up, you need to generate and fetch a token into your application. This tutorial shows you how to do this with Unity.
Fetching the Token
You need to determine the full URL to reach your token service. In my example, the service is running on the local machine, which is why I’m looking at "http://localhost:8080/..."
. I’m also using my-channel as the channel name and 0 as the userId.
The example found in the Agora-Unity-Quickstart project calls a method found in tools/RequestToken.cs:
The FetchToken method above makes a request to the token server and passes the response as a string into the callback method. The callback method allows greater control of how to handle the received response. For example, in one instance you might need to connect to the channel. Or you might use the method within the OnTokenPrivilegeWillExpireHandler event to update the token for a channel you’re already connected to.
I’m using UnityWebRequest for the GET request. For information on this class, see Unity’s documentation on UnityWebRequest.Get.
Connecting to a Channel
Connecting to a channel with a token in Unity uses the JoinChannelByKey method, like so:
RtcEngine.JoinChannelByKey(channelToken, channelName, "", 0);
Staying Connected
Tokens have an expiration by design. Once a user’s token expires, they are removed from the channel. Using the example token server, the token lifetime can be set as a default value by the token server or as a parameter sent in the request.
Once the user connects with the token, they will need to keep that token updated to remain in the channel. In order to have an uninterrupted session in the channel, the OnTokenPrivilegeWillExpireHandler is called 30 seconds before a token is set to expire. From there you can contact the token server again and request an updated token. The returned token can then update the current status of the user with IRtcEngine.RenewToken().
Here’s an example of the token expiring handler and RenewToken being used in C#:
Testing
Full Example
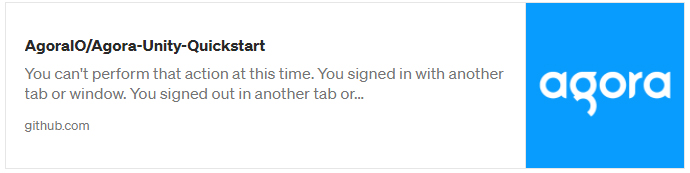
Other Resources
For more information about Agora applications, take a look at the Agora Video Call Quickstart Guide and Agora API Reference.
I also invite you to join the Agora Developer Slack community.