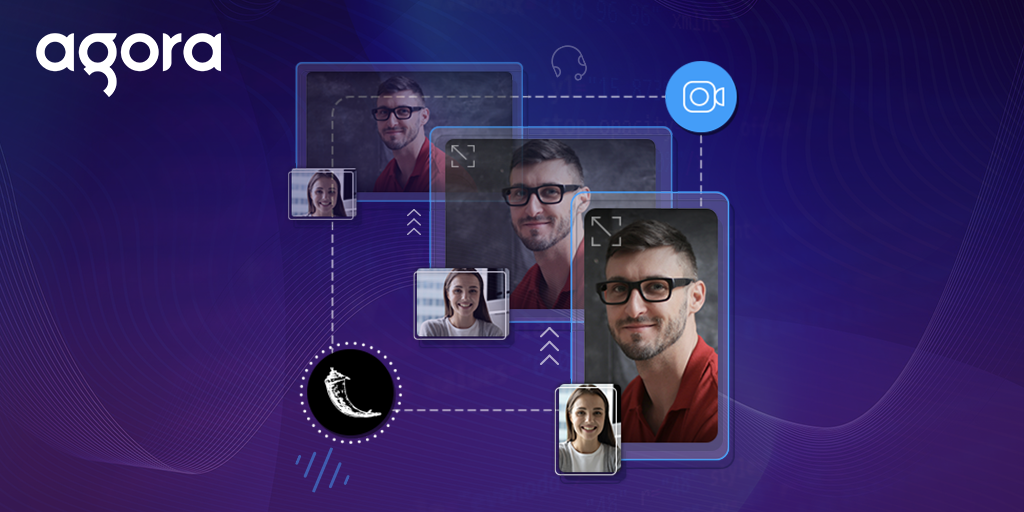
Build a Scalable Video Chat App with Agora in Flask
Author: Kofi Ocran. I am a software engineer who builds products and services with web technologies. I occupy the sweet spot between software engineering and data science and learn more by sharing my knowledge through technical articles.
Introduction
Flask is a micro web framework written in Python. It is mostly used to build API endpoints, but it can be extended to build fully fledged applications. We are going to build a one-on-one video calling application with Flask using the hassle-free Real-Time Engagement platform with Agora.
Previously, I built a video chat app with WebRTC and Laravel and wrote a blog about it here: Adding Video Chat To Your Laravel App. WebRTC is one of the ways through which you can implement video chat features. Companies like Agora provide a fully packaged video chat SDK to provide a high-quality Real-Time Engagement video chat experience. As someone who has WebRTC development experience, I can tell you there are some limitations with WebRTC, such as:
- Quality of experience: Since WebRTC is transmitted over the Internet, which is a public domain, the quality of experience is hard to guarantee.
- Scalability: Scalability is fairly limited on group video calls due to the peer-to-peer nature of WebRTC.
After my experience with the Agora platform, I concluded it was not worthwhile for a company to invest a lot of development hours into building a video call feature as part of their main application with WebRTC. Agora will save you a lot of development hours. Through this article, I’m going to show you how to quickly build a video call application in Flask with Agora.
Why Agora Is the Preferred Solution
After building a video chat app with Agora, I want to highlight some of the advantages:
- There’s one SDK for everything — voice, video, live streaming, screen sharing, and so on.
- I didn’t have to set up a turn server with coturn on Amazon EC2 as I did in the other implementation to relay traffic between peers on different networks.
- You get 10,000 minutes every month free, and this gives you the flexibility to develop your solution prototype for free.
- You don’t have the challenge of managing the underlying infrastructure supporting the video call functionality.
- Intuitive API documentation is available.
Prerequisites
- Python 3.8.5
- A Flask application with authentication. Download the following starter project to get started: Flask Auth Starter Project. We are going to build on top of this starter project.
- A fair understanding of the factory and blueprint pattern in Flask. The starter project has been structured that way.
- A free Pusher account on Pusher.com.
- An understanding of Pusher presence channels and the Python server library.
- An Agora developer account (see How to Get Started with Agora).
Project Setup
1. Create and activate a Python 3 virtual environment for this project.
2. Open your terminal or command prompt and navigate to the starter project you downloaded as part of the prerequisites. The folder is named agora-flask-starter.
3. Follow the instructions in the README.md file in the agora-flask-starter folder to set up the application.
4. Install additional dependencies from your terminal or command prompt:
pip install pusher
5. Download the AgoraDynamicKey Python 3 code from the Agora repository: AgoraDynamicKey
Keep the downloaded folder in a location outside the project folder. Some of the files in the folder will be copied into our project when we’re configuring the back end.
Configuring the Back end
We are going to create the views for the Agora blueprint and import the classes needed to generate the Agora token to establish a call. We will set up Pusher at the server-side as well.
1. Add the downloaded AgoraDynamicKey generator files.
- Open your terminal or command prompt and navigate to the Agora blueprint directory:
cd app/agora
- Create a subdirectory named agora_key:
mkdir agora_key
- Copy AccessToken.py and RtcTokenBuilder.py from the src directory in the downloaded files and add them to the agora_key directory.
2. Create the Agora view.
Add the following code to the views.py file in the app/agora directory:
Breakdown of Methods in agora/views.py
- index: To view the video call page. Only authenticated users can view the page. Non-authenticated users are redirected to the login page. We return a list of all the registered users.
- pusher_auth: It serves as the endpoint for authenticating the logged-in user as they join the Pusher presence channel. The ID and name of the user are returned after successful authentication with the pusher.
- generate_agora_token: To generate the Agora dynamic token. A token is used to authenticate app users when they join the Agora channel to establish a call.
- call_user: This triggers a make-agora-call event on the presence-online-channel to which all logged-in users are subscribed.
The data broadcast with the make-agora-call event across the presence-online-channel contains the following:
- userToCall: This is the ID of the user who is supposed to receive a call from a caller.
- channelName: This is the call channel that the caller has already joined on the front end. This is a channel created with the Agora SDK on the client side. It is the room the caller has already joined, waiting for the callee to also join to establish a call connection.
- from: The ID of the caller.
Using the make-agora-call event, a user can determine whether they are being called when the userToCall value matches their ID. We show an incoming call notification with a button to accept the call. They know who the caller is by the value of from.
Configuring the Front End
We are going to create the user interface for making and receiving the video call with the ability to toggle the on and off states of the camera and the microphone.
1. Update the HTML file for the index view.
The HTML file will contain the links to the CDN for Agora SDK, Vue.js, Pusher, Bootstrap for styling, and our custom CSS and JavaScript.
The index.html file will also inherit a base template, which is used to render the view:
- Open the index.html file in the agora subdirectory in the templates directory. The location is app/templates/agora.
Currently, it only displays a welcome message for an authenticated user. - Update the index.html file with the following code:
We use Flask’s templating language to be able to reuse some code. As indicated earlier, we inherit a base template named base.html. It has the following blocks:
- head_scripts: This is the block where we place the link to the Agora SDK and our index.css for styling the video call page.
- content: The content block contains the user interface for rendering the video stream with its control buttons.
- bottom_scripts: This block contains the links to the Pusher SDK, an instance of the Pusher Class with the presence channel authentication, Axios for sending AJAX requests, and Vue.js for writing the client-side logic for our video chat application.
2. Create the static files
We have index.css for custom styling and index.js, which is our script for handling the call logic.
Run the following command to create the files from your terminal or command prompt:
cd static/agora/
touch index.css index.js
Add the following to index.js
Add the following to index.css:
Breakdown of the Agora Call Page
On the video call page (app/templates/agora/index.html), we display buttons that bear the name of each registered user and whether they are online or offline at the moment.
To place a call, we click the button of a user with online status. An online user is a user who is available to receive a call. For our demo, we see a list of users. The user named Bar is indicated as being online. The caller named Foo can call Bar by clicking the button:
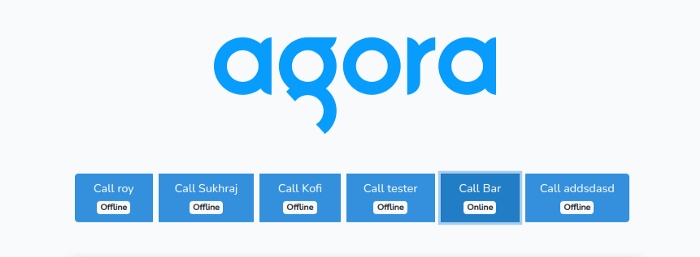
Bar gets an incoming call notification with Accept and Decline buttons and the name of the caller:
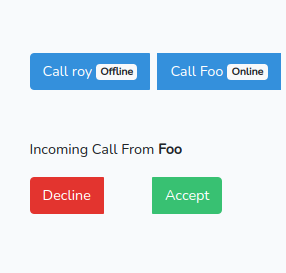
From the call notification image above, we see that the caller’s name is Foo. Bar can then accept the call for a connection to be established.
The following diagram explains the call logic in terms of the code:

3. Update flaskenv variables with Pusher and Agora keys
The .flaskenv file is located at the root of your project folder. Add the credentials you got from Agora and Pusher. Add the secret key too if you didn’t add it during the project setup:
Testing
1. Start the Flask development server from your terminal.
flask run
2. Open two different browsers or two instances of the same browser, with one instance in incognito mode, and navigate to the registration page: http://127.0.0.1:5000/register.
3. In one of the browsers create four users by registering four times.
4. Login with the account details you just created on each of the browsers from the login page: http://127.0.0.1:5000/login.
5. In each of the browsers you opened, the other users registered on the application are displayed.
6. In one browser, you can call an online user by clicking the button that bears their name.
7. The other user is prompted to click the Accept button to fully establish the call.
Video Demonstration of the Video Call
To confirm that your demo is functioning properly, see my demo video as an example of how the finished project should look and function: Agora + Flask Video Call Demo
Conclusion
You have now implemented the video call feature in your Flask application! It’s not that hard, right?
To include video calling functionality in your web app, you don’t have to build it from scratch.
Agora provides a lot of great features out of the box. It also helps businesses save development hours when implementing video chat into existing projects. The only thing a developer has to do is build a compelling front end — Agora handles the video chat back end.
Online Demo link: https://watermark-remover.herokuapp.com/auth/login?next=%2Fagora
Completed Project Repository (it is located on the branch named completed in the starter kit):
https://github.com/Mupati/agora-flask-starter/tree/completed
Make sure the demo link or production version is served over HTTPS.
Test accounts:
foo@example.com: DY6m7feJtbnx3ud
bar@example.com: Me3tm5reQpWcn3Q
Other Resources
- Available events on the Agora Client
- For more information about Agora.io applications, take a look at the Agora Quickstart Guides
- Take a look at the complete documentation for the functions discussed above and many more: Agora Web SDK API
And I invite you to join the Agora Developer Slack Community.