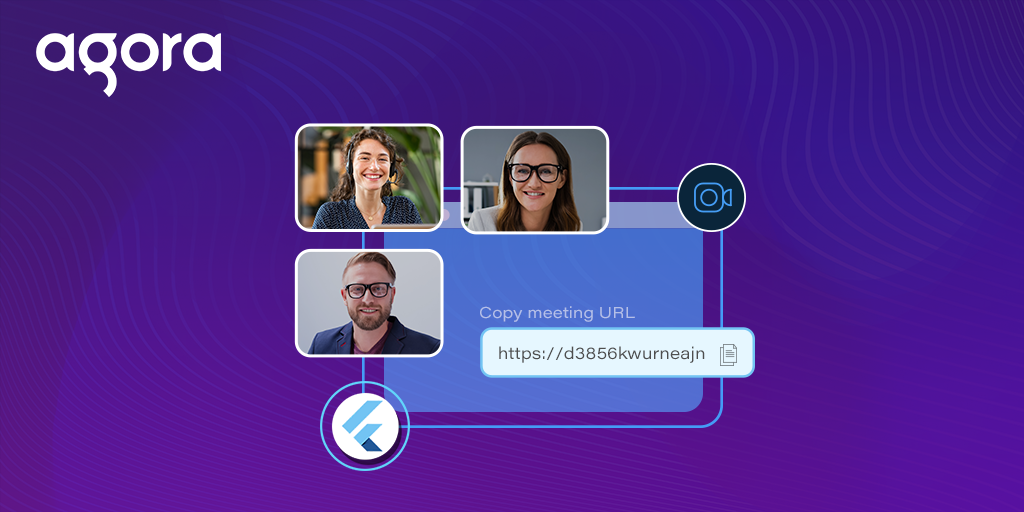
Adding Meeting URLs to your Agora Live Video Call using the Flutter UIKit
When making an application such as one for video calls, you may want to share links to let your users’ friends jump into the same video call or livestream that they’re watching. The second-best way to achieve this is by sharing a room code for people to type into their version of the app. But a much better option is to share a link that opens the app right to the place you want. That is where we can use universal links.
What are Universal Links?
Often, if there is an equivalent web page for a view that exists in an app. For example, if you’re viewing an Instagram profile on your phone’s web browser, there is also a way to show the same content in the Instagram app. Universal links are the gateway for going from the web browser content to the app’s equivalent content.
In some cases, universal links can’t have a web equivalent; for example, a complex game that will not run in a web browser. A universal link in that scenario could lead to a template page, which can then be passed through to your application to render the full content or to join the game.
Universal links must be set up individually on Android and iOS in order to open the app directly using the URL.
Prerequisites
- An Agora developer account — Sign up here
- VS Code or Android Studio
- Xcode 12.3 or later
- A physical Android and iOS device
- A public server with an SSL certificate (HTTPS)
Setting Up Universal Links
Setting up universal links has been made easier in recent years, but it still has two main steps:
- Setting Up the Website
- Setting Up the App
Let’s start with the website side.
Setting Up the Website
In Flutter, we will have to create two reference points for the URL to open the app-based links on the platform that we are using: Android or iOS.
Let’s go over both platforms and see how we can set up the website to enable universal links.
Android
For Android, we begin by declaring the association of our website to our application by creating a JSON
file saved at .well-known/assetlinks.json.
In the JSON
file, add the following attributes:
[{
"relation": ["delegate_permission/common.handle_all_urls"],
"target": {
"namespace": "agora_uikit",
"package_name": "io.agora.agora_uikit_example",
"sha256_cert_fingerprints": [""]
}
}]
namespace
: The name of your Flutter application.package_name
: The application ID declared in the app’sbuild.gradle
file.sha256_cert_fingerprints
: You can use the following command to generate the fingerprint via the Java keytool:
keytool -list -v -keystore my-release-key.keystore
iOS
For iOS, we will do something similar by creating a file named apple-app-site-association
. Note that the file is saved without any extension.
Apple App Site Association (AASA) should be served from the root. Add appropriate headers to your CDN to ensure that this file is served with the Content-Type=application/pkcs7-mime
header. An AASA file looks something like this:
{
"applinks": {
"apps": [],
"details": [{
"appIDs": ["DEVELOPMENT_TEAM.PRODUCT_BUNDLE_IDENTIFIER"],
"components": [
{
"/": "*"
}
]
}]
}
}
You just need to replace DEVELOPMENT_TEAM
and PRODUCT_BUNDLE_IDENTIFIER
with the associated values from your app. To find them, you can run a couple of commands in a terminal from your the iOS directory inside your project, as in this example:
grep -r -m 1 "DEVELOPMENT_TEAM" .; grep -r -m 1 "PRODUCT_BUNDLE_IDENTIFIER" .
Your terminal will look similar to this:
=> grep -r -m 1 "DEVELOPMENT_TEAM" .; grep -r -m 1 "PRODUCT_BUNDLE_IDENTIFIER" ../AppName.xcodeproj/project.pbxproj: DEVELOPMENT_TEAM = 278494H572;
./AppName.xcodeproj/project.pbxproj: PRODUCT_BUNDLE_IDENTIFIER = io.agora.AppName;
In this case, the values we want are 278494H572
and io.agora.AppName
, respectively.
To check that your website is set up correctly, head to Branch.io’s validator.
More examples can be found in Apple’s documentation here.
Once you have added AASA, your server directory should look something like this:
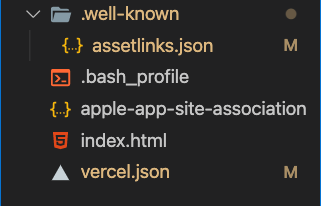
Now that that’s set up, let’s get to the app portion of setting up the meeting URLs.
Setting Up the App
Your app needs to establish trust that your app has been approved by the website to automatically open links for that domain. If the system successfully verifies that you own the URL, the system automatically routes those URL intents to your app.
Let’s go over the process for both Android and iOS and see how we can set up that trust between the URL and our app.
Android
For Android, we simply have to add intent filters to our AndroidManifest.xml
:
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="https"
android:host="[YOUR_HOST]" />
</intent-filter>
In this code replace [YOUR_HOST]
with your HTTPS server.
iOS
The same process can be done for iOS simply by adding Associated Domains compatibility to your project using Xcode. To do this, open the iOS directory of your Flutter application on Xcode and select Runner
:
- In Runner, select Signing & Capabilities.

- Click+Capability
- From the list, select Associated Domains.
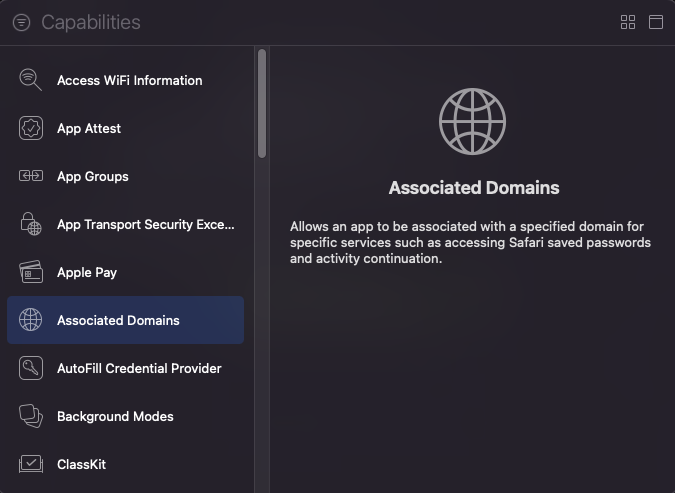
- Click the + icon and add your server URL:
applinks:www.link-to-your-server.com
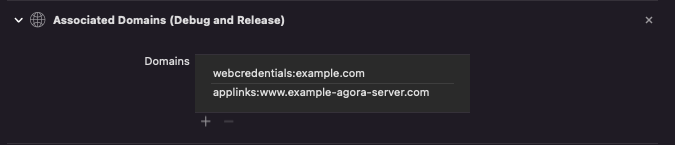
And with that, we are all set. Let’s see how we can use this in our UIKit to share invites.
Agora Flutter UIKit
The Flutter UIKit is now available with universal link support. This support helps you automatically share the invite messages and to verify the trust between your URL and your application.
To use it, you can navigate to the deep link branch of the Flutter UIKit: https://github.com/AgoraIO-Community/Flutter-UIKit/tree/deeplink
To let the Flutter UIKit help you in generating the invite message and the meeting URL simply add deeplinkBaseUrl
to your AgoraClient
such that you have the client object declared, as here:
final AgoraClient client = AgoraClient(
agoraConnectionData: AgoraConnectionData(
appId: "<--Add your APP ID here-->",
channelName: "test",
),
enabledPermission: [Permission.camera,Permission.microphone],
deepLinkBaseUrl: "link-to-your-server.com",
);
Let’s go ahead and see how it looks.
Testing
You can test this out on both platforms: Android and iOS. From the root directory enter this command in your terminal:
flutter run
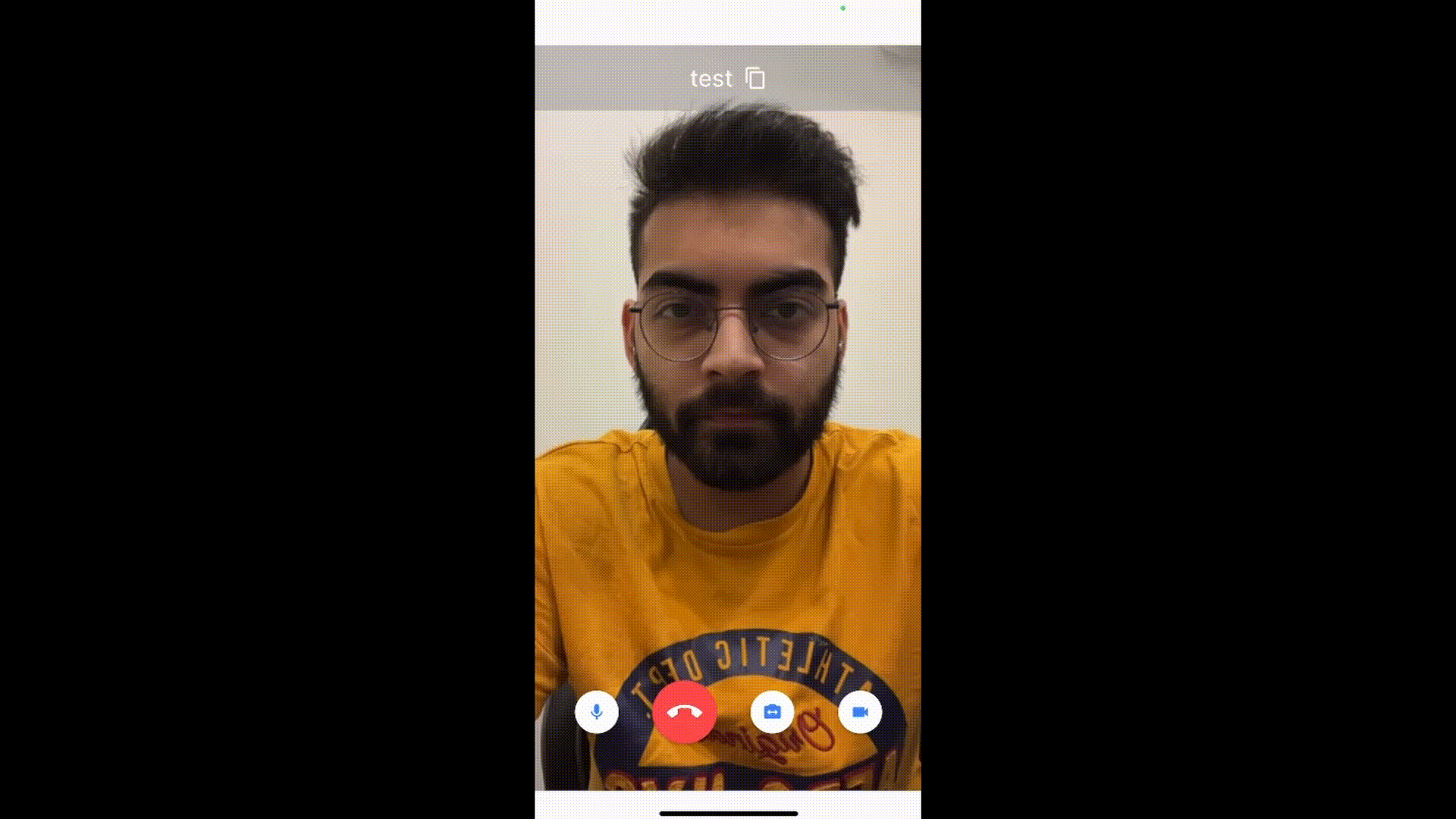
Conclusion
You now have a video chat application through which you can share meeting URLs and invite others to join your call directly without having to enter the channel name.
To use universal links with your Agora Flutter UIKit, have a look at the complete code here.
Other Resources
To learn more about the Agora Flutter SDK and other use cases, see the developer guide here.
You can also have a look at the complete documentation for the functions discussed above and many more here.
And I invite you to join the Agora.io Developer Slack Community.