Note: Updated for use with NG SDK (4.x)
Integrating video streaming features into your application can be very tedious and time-consuming. Maintaining a low-latency video server, load balancing, and listening to end-user events (screen off, reload, etc.) are some of the really painful hassles — not to mention having cross-platform compatibility.
Feeling dizzy already? Fear not! The Agora Video SDK allows you to embed video calling features into your application within minutes. In addition, all the video server details are abstracted away.
In this tutorial, we’ll create a bare-bones web application with video calling features using vanilla JavaScript and the Agora Web NG SDK.
You can test a live demo of this tutorial here.
Prerequisites
- Google Chrome
- You can get started with Agora by following this guide and retrieve the Appid.
- Also, retrieve the temporary token if you have enabled the app certificate.
Project Setup
This is the structure of the application that we are developing:
Building index.html
The structure of the application is very straightforward.
You can download the latest version of the Agora Web SDK from the Agora Downloads page. Or you can use the CDN version instead, as shown in the tutorial:
There is a container with an id me
that is supposed to contain the video stream of the local user (you).
There is a container with an id remote-container
to render the video feeds of the other remote users in the channel.
Building styles.css
Now, let’s add some basic styling to our app:
Building the Video calling client
First, let’s make some helper functions to handle trivial DOM operations like adding and removing video containers:
The architecture of an Agora Video Call
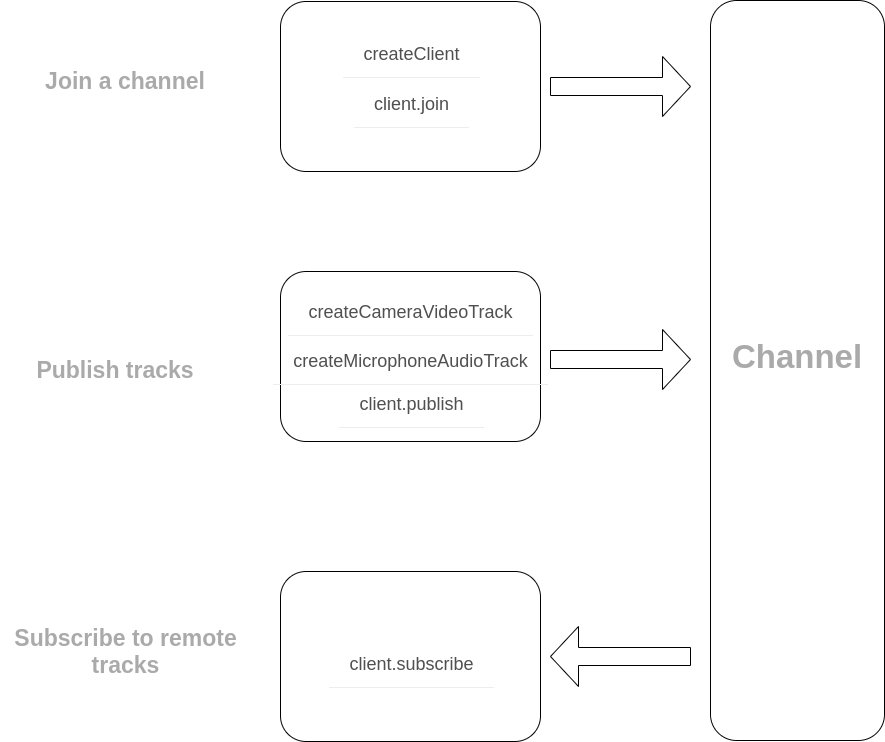
So what’s the diagram all about? Let’s break it down a bit.
Channels are similar to chat rooms, and every App ID can spawn multiple channels.
Users can join and leave a channel at will.
We’ll be implementing the methods mentioned in the diagram in script.js
.
Creating a Client
First, we need to create a client object by calling the AgoraRTC.createClient method. Pass in the parameters to set the video encoding and decoding format (vp8) and the channel mode (rtc):
Creating Local Media Tracks and Initializing
Let’s create audio and video track objects for the local user by calling the AgoraRTC.createMicrophoneAndCameraTracks
method. (Although optional, You can also pass in the appropriate parameters as per docs to tweak your tracks).
We can now initialize the stop button and play the local video feedback in the browser (“me” container) to provide feedback:
initStop
is defined at the end of the code as a cleanup function:
Adding Event Listeners
To display the remote users in the channel and to handle the view appropriately when somebody enters and exits the video call, we’ll set up event listeners and handlers:
Joining a Channel
Now we’re ready to join a channel by using the client.join
method:
If you have the app certificate enabled in your project, you have to generate a temporary token and pass it into the HTML form. If the app certificate is disabled, you can leave the token field blank.
For production environments:
Note:This guide does not implement token authentication, which is recommended for all RTE apps running in production environments. For more information about token-based authentication in the Agora platform, see this guide: https://bit.ly/3sNiFRs
We will allow Agora to dynamically assign a user ID for each user who joins in this demo, so pass in null
for the UID parameter.
Publishing Local Tracks into the Channel
Now it’s time to publish our video feed on the channel:
Testing the project
Run the project by double-clicking the index.html. Make sure you have the latest version of chrome for testing the project.
Conclusion
Shazam! Now we can conduct a successful video call in our application.
Note: When you try to deploy this web app (or any other that uses the Agora SDK), make sure the server has an SSL certificate (HTTPS connection).
The codebase for this tutorial is available on GitHub.
Other Resources
For more information about building applications using Agora SDKs, take a look at Agora Video Call Quickstart Guide and Agora API Reference.
I also invite you to join the Agora Developer Slack community.