
Joining Multiple Agora Channels in Unity
Hello, brave developers! In this tutorial you’ll set up multiple Agora channels in a Unity 2019.4.1 (LTS) environment. This project will demonstrate how to join multiple Agora channels via AgoraChannel objects. In this example, you’ll encounter the intricacies of joining multiple channels and publishing your stream to one allowed channel.
Getting Started with Agora
To get started, you need an Agora account. If you don’t have one, here is a guide to setting up an account.
This project builds on the Unity Multichannel demo. It uses a basic Unity scene and the Agora Video SDK.
UI Setup
First, you create the panel prefab to house the video feeds rendered to VideoSurface objects, which are managed by the callbacks as users join and leave the channels. Then, you create the buttons that enable the Agora functionality:
- Join: Join the channel and see other people who are publishing their stream.
- Leave: Disconnect from the channel stream.
- Publish: Publish your video stream into the channel for others to see.
- Unpublish: Remove your stream from the channel while remaining in the channel to see others.
Right-click in the Hierarchy panel and select UI > Scroll View. Deselect the Horizontal attribute in the ScrollRect component, and delete the vertical and horizontal scroll bars. Set the RectTransform attributes as follows: Left 250, Right -300, Top -140, Bottom -140. Make sure the Viewport child RectTransform is zeroed out. Set the Content child Height to 300. Right-click Content and select Create Empty to make another child, and name it SpawnPoint. Set the position and anchor pivots like so:

Next, create four buttons named JoinPartyButton, LeavePartyButton, PublishToPartyButton, and UnpublishFromPartyButton. Update the text in the buttons to read “Join Party,” “Leave Party,” “Publish,” and “Unpublish.”
In the Project window, make a folder named Prefabs, and drag the entire panel into the folder to create a reusable prefab object.
Drag another prefab into the scene to make another channel panel, like so:
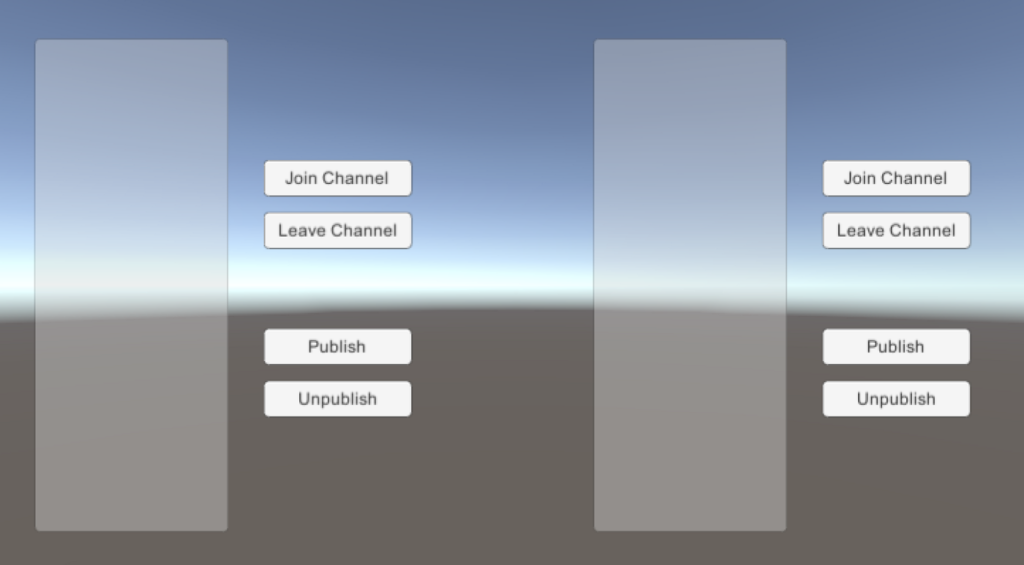
Agora Engine Script
Create an empty object in the scene and name it AgoraEngine. Create a script with the same name and attach it to the newly created object:
Be sure to fill out your App ID!
Channel Panel Script
Next, create a script named ChannelPanel.cs. First, you create the callbacks that listen for events from the Agora engine. Next, you create the code to manage the video panels. As users enter, exit, and publish to channels, their video surfaces create and destroy accordingly.
Initialization
Video Surface Management
Here, you write the code to instantiate and remove video surfaces that render the video streams from each user. The video surfaces spawn into an adjustable UI panel that allows you to scroll through the active video feeds. Pay attention to the code to understand how to appropriately adjust the length of the UI panel as users join and leave.
Callbacks
The callbacks are functions that automatically fire during certain Agora events, like joining and leaving a channel. With the callbacks, you define your own method, subscribe to the callback, and handle the event however you’d like.
Most of the callbacks in this demo are pretty standard, but notice the OnRemoteVideoStatsHandler. The remote video stat handler is used to determine whether or not a player is publishing their video stream.
Note: When using the AgoraChannel object, the users behave like a Broadcasting channel profile. Joining a channel doesn’t mean you automatically publish your video feed, too, as in Communication mode. In this example, we have to join a channel and publish our stream in order for others in the channel to see and hear us.
The remote video stats determine if the video surface should be added or removed based on the data they are sending across the network. If their data bitrate is zero, they aren’t publishing and their video surface should be removed. If their bitrate is greater than zero, they are publishing and their video should be displayed.
Button Functionality
Drag the corresponding functions into the OnClick() events in the Inspector window for the buttons:

I like to prefix my button functions with “Button_” so that I can easily find the functions in the editor and they are all grouped together for easy access.
Prefab Setup
To attach the script to the prefab, select the ChannelOne prefab instance in your scene, navigate to the Inspector panel, and click the Open button.
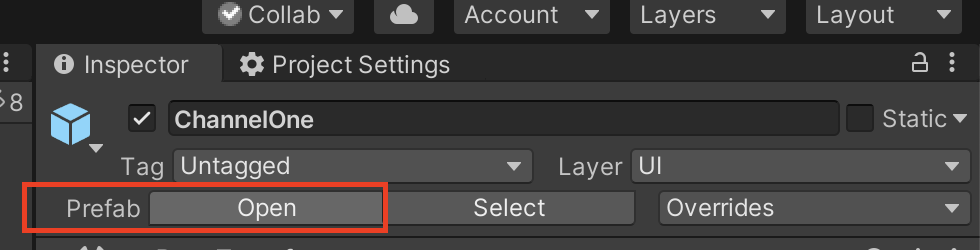
In the view of your open prefab, drag and drop the script into the ChannelPanel object. Drag the SpawnPoint child object into the Video Spawn Point field and the Content child object into the Panel Content Window field. Then click the back arrow to return to your scene.

Notice that both prefabs now have the script attached with their respective child objects assigned in the Inspector. The Channel Name and Channel Token fields are blank because each needs a unique value generated from the Agora Console.
Token Creation
By this point, you should have two successfully created prefabs with no compile issues in your scene like so:
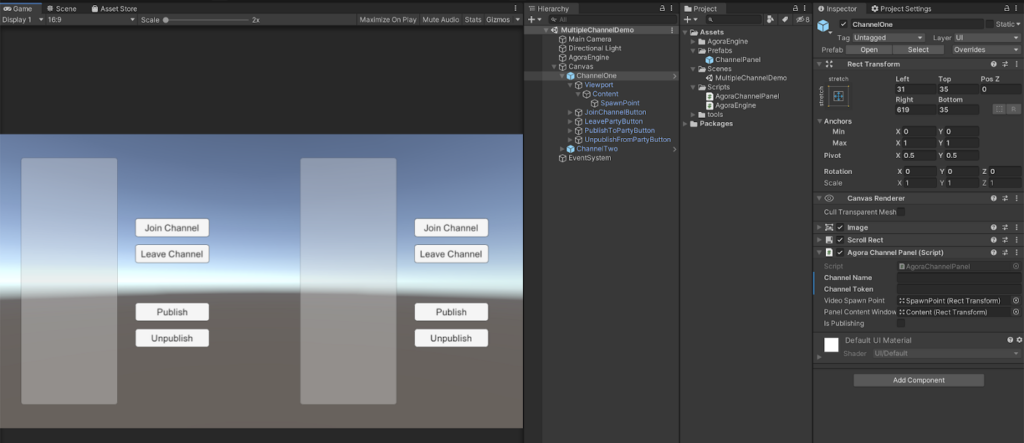
(If you are having issues, you can find the full code and project below.)
Next, you need to generate temporary tokens from the Agora console, insert them into the respective ChannelName and ChannelToken fields for each channel prefab, and then test:
- Go to console.agora.io.
- Navigate to the Project Management tab on the left sidebar.
- Click the Create button.
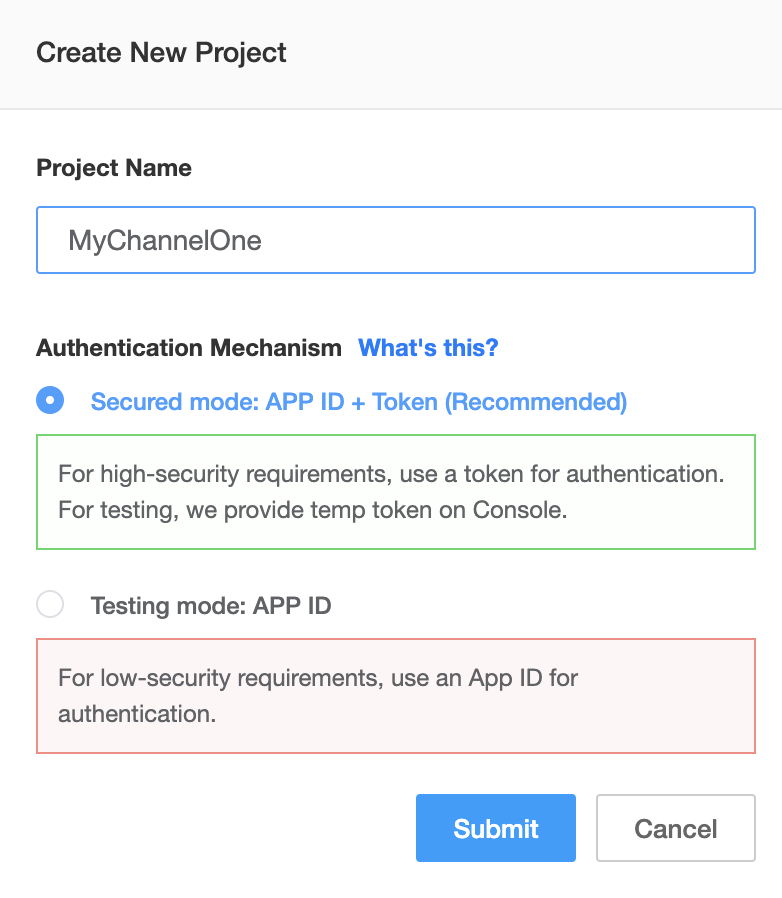
- Select Secured mode, name the project whatever you’d like, and click the Submit button.
- Your new project pops into view like so. Next, press the key that reads “Temp token for video call.”

- Input a channel name. (I use ChannelOne and ChannelTwo for my demo) and click the Generate Temp Token button.
- Copy the values from here:

- To here:

Repeat these steps for ChannelTwo.
Conclusion
Now it’s time to test! Remember, you have to publish your stream for other users to see you in the channel. You can publish to only one channel concurrently, but you can view the streams of many channels at a time.
If you learned something, post it in the #unity-help-me slack channel and direct message me. And be sure to teach someone else!
You can find the entire project here on Agora’s community GitHub if you’d like to download the project for yourself.
Cheers!
– Joel