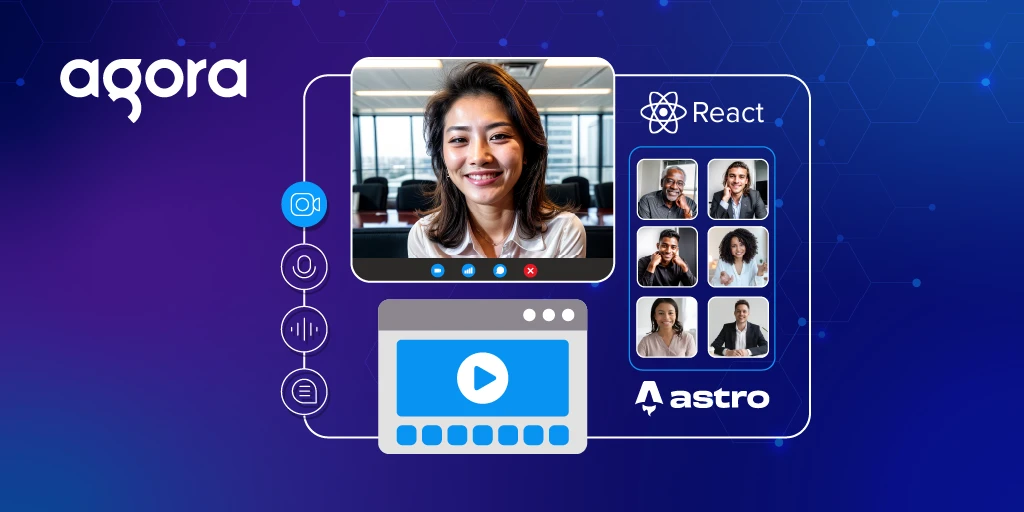
Build a Video Call App with Astro and ReactJS
Astro is a framework that renders static HTML to your website with no JavaScript unless you explicitly ask for it and it is gaining popularity fast. But can you add video calls to your Astro website?
The answer is yes! This article will teach you exactly how to do it.
What We Will Use
Of course, we need the Astro framework, since it is the title of this article.
We will use Agora for the video call portion of the app. Specifically, we will use the agora-rtc-react
package. This package is a React SDK for interfacing with Agora.
Currently, there is no native Astro package. But Astro was built to be used with almost any popular web framework. So the agora-rtc-react
package will work just fine.
We will use Tailwind CSS for minor styling of the application.
Create an Agora Account
Sign up for an Agora account, and log in to the dashboard.
Navigate to the Project List tab under the Project Management tab. Create a project by clicking the blue Create button.
Retrieve the App ID, which we’ll use to authorize the app requests as we develop the application.
Initialize the Astro Project
- Create your Astro project by using
npm create astro@latest
. - Add Tailwind CSS by using
npm astro add tailwind
. - Add React by using
npm astro add react
. - Add the Agora UI Kit by using
npm install agora-rtc-react
. - Add
PUBLIC_AGORA_APP_ID = '<---Your App Id--->'
to your.env
file.
Structure of the Project
Building a Form with Astro
Astro SSG vs SSR
By default, Astro projects use a static site generator (SSG) that creates a static site which you cannot change. Astro provides a way to interface with various frameworks (React, Svelte, Vue, etc.) using a mechanism called islands. Forms need to be interactive, and we could use an island to get this done. However, you can create a form with Astro that ships with zero JavaScript.
To do that, we need to enable the server-side renderer (SSR) by adding output: 'server'
to the astro.config.mjs
file. This means that our pages will get rendered on the server. This brings the benefits of being able to access server-side data and to run some functions before the page loads.
Form UI
For the case of creating and submitting a form, we will load the page initially to display the form. Then whenever we submit the form, it will act much like an API endpoint.
Normally, when you submit a form the data is added to the URL, and you would have to parse it out. By changing the form’s data transfer method to POST, it will send the form data as part of the Request
body. For this application, the only data we need is the name of the channel the user is trying to join:
Our form should look like this:
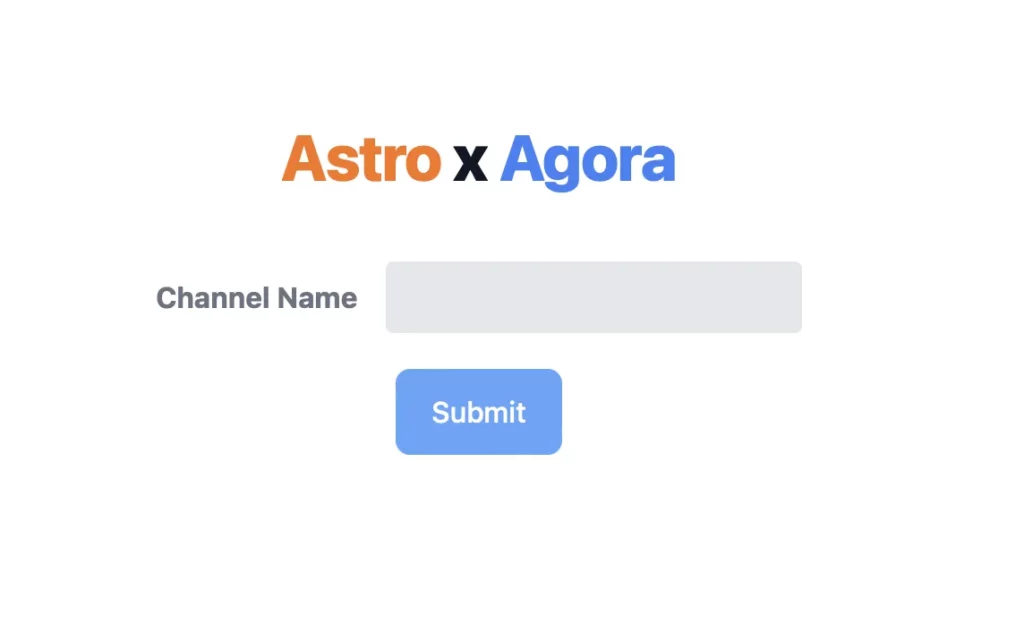
Retrieve Form Data and Redirect to the Call Page
Once the form is submitted with the data in the Request
, the page is reloaded and the data is sent to the server. This data can be retrieved server-side and acted on before the page is displayed. In this case, we will check for errors. If we find any, we will render the same page again to show the user the error. If there is no error, we will redirect the user to the page associated with the video call channel they entered:
Call Page
In the Call component that we will build with the agora-rtc-react
, we want to print the name of the channel we have joined.
The redirect we used in the previous section is dynamic, and it will be different depending on the channelName we entered. To handle this in Astro, we need to create a channel
folder and in the folder define a file named [channelName].astro
. The square brackets signify that the dynamic ${channelName}
in our URL will be passed as a parameter in this component. We just need to retrieve the channel name and display it in our UI.
client:only
Directive
For our Call component we pass the channelName
and the appId
. But we also need to add a client:only="react"
directive. This directive is needed for creating an Astro island. As mentioned, Astro generates static HTML code for the site, and the video call that we create needs to be interactive.
So without this directive Astro will try to render static HTML from this React component. However, by using client:only
we skip the server-side rendering, and just let React do what React wants to do: render only on the client.
Since Astro supports many frameworks we also need to specify which framework it needs to use:
Add the Call Component
This last component is a pure React component. The Call component will contain three key components:
- Videos of all the participants
- Channel name
- End call button
The channel name is a simple text at the top left of the screen. The call button is at the bottom middle of the screen. Whenever you click the button, it returns you to the previous screen, where you can join a different video call.
The interesting part is displaying the videos of all the participants. In order to do that we need to create an AgoraRTCProvider
, which initializes and gives us access to the Agora RTC service. Inside this we can now display the video:
Videos
The Videos component will be the part of the site that displays the videos of all the participants. Many hooks are used to set up the call:
- useLocalMicrophoneTrack() retrieves the current user’s microphone.
- useLocalCameraTrack() retrieves the current user’s video input.
- useRemoteUsers() retrieves all the user information for the remote users.
- useRemoteAudioTracks() retrieves the audio for those users.
- usePublish() publishes the current user’s video and audio.
- useJoin() joins the channel for the video call.
Then we need to make sure all the audio for the remote users is started: audioTracks.map((track) => track.play());
Finally, we need to define our UI: first a loading state, while we wait for the local user’s microphone and video to begin, and then a grid of all the users who are in the call:
Our final video call should look like this:

Summary
With that, we have a complete video call experience. Here’s how we built it:
- Create our Astro project.
- Install Tailwind CSS, React, and the Agora SDK.
- Create a form to input the channel name.
- Redirect the site to a URL with the channel name.
- Display the channel name with a video call.
The code for this project can be found here. You can find out more about Agora video calling here.
Thank you for reading!